The SMB library allows you to access Microsoft Windows Network file system.
You can read more information about this protocol here: Server Message Block - Wikipedia, the free encyclopedia
SMB can be used to upload or download files from Windows shared folders.
This library wraps JCIFS library: JCIFS
Before starting with your own solution it is recommended to download an existing SMB client such as AndSMB: https://play.google.com/store/apps/details?id=lysesoft.andsmb and get it working. There are several configurations that may prevent a SMB client from working properly.
If you are using Windows 7 you may want to allow access without an existing Windows account:
Go to Advanced Sharing Settings and choose Turn off password protection.
As with any network operations, all the operations are done in the background. An event will be raised when the operation completes.
For example to list all files and directories we can use a code such as:
The Url can include all kinds of parameters. See this page for more information: JCIFS API
The library is available here: http://www.b4x.com/forum/additional-libraries-official-updates/17180-smb-library.html
You can read more information about this protocol here: Server Message Block - Wikipedia, the free encyclopedia
SMB can be used to upload or download files from Windows shared folders.
This library wraps JCIFS library: JCIFS
Before starting with your own solution it is recommended to download an existing SMB client such as AndSMB: https://play.google.com/store/apps/details?id=lysesoft.andsmb and get it working. There are several configurations that may prevent a SMB client from working properly.
If you are using Windows 7 you may want to allow access without an existing Windows account:
Go to Advanced Sharing Settings and choose Turn off password protection.
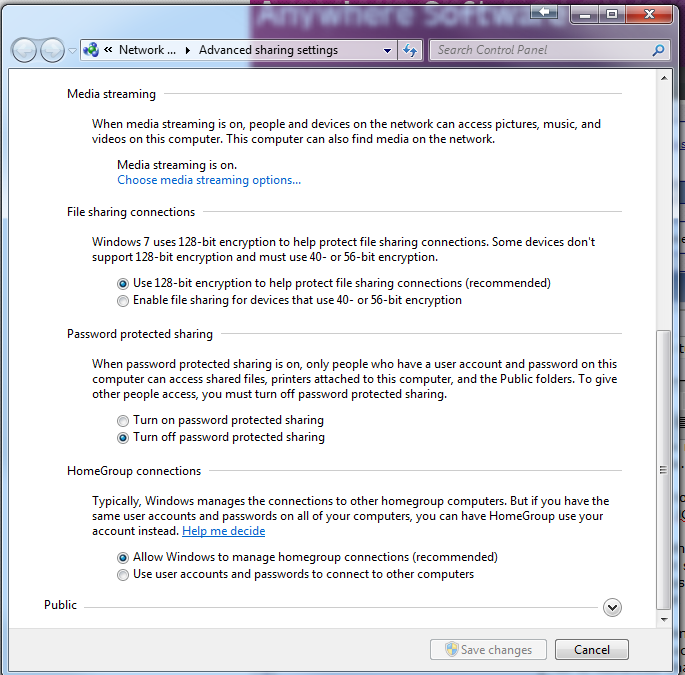
As with any network operations, all the operations are done in the background. An event will be raised when the operation completes.
For example to list all files and directories we can use a code such as:
B4X:
Sub Process_Globals
Dim SMB1 As SMB
End Sub
Sub Globals
End Sub
Sub Activity_Create(FirstTime As Boolean)
If FirstTime Then
SMB1.Initialize("SMB1")
End If
SMB1.ListFiles("smb://USER-PC/Users/Public/", "")
End Sub
Sub SMB1_ListCompleted(Url As String, Success As Boolean, Entries() As SMBFile)
If Not(Success) Then
Log(LastException)
Else
For i = 0 To Entries.Length - 1
Log("*****************")
Log(Entries(i).Name)
Log(Entries(i).Directory)
Log(DateTime.Date(Entries(i).LastModified))
Log(Entries(i).Parent)
Log(Entries(i).Size)
Next
End If
End Sub
The Url can include all kinds of parameters. See this page for more information: JCIFS API
The library is available here: http://www.b4x.com/forum/additional-libraries-official-updates/17180-smb-library.html