The attached class is an implementation of a MJPEG over Http decoder.
The data is a mix of strings and raw bytes.
It is tempting to convert the bytes to a string. However raw bytes do not represent a valid string so you can lose important information during the conversion (which will also slow down the program).
Edit: For new projects it is recommended to use BytesBuilder.
The following sub is used to search for strings or bytes in the raw bytes directly:
The data is copied to a large array. Once a frame is found it is parsed and the array is trimmed using ByteConverter.ArrayCopy.
It is compatible with B4i, B4A and B4J.
It works better in release mode.
You will need to handle disconnections.
The data is a mix of strings and raw bytes.
It is tempting to convert the bytes to a string. However raw bytes do not represent a valid string so you can lose important information during the conversion (which will also slow down the program).
Edit: For new projects it is recommended to use BytesBuilder.
The following sub is used to search for strings or bytes in the raw bytes directly:
B4X:
Private Sub IndexOfString(s As String, Start As Int) As Int
Return IndexOf(s.GetBytes("ASCII"), Start)
End Sub
Private Sub IndexOf(bs() As Byte, Start As Int) As Int
For i = Start To index - 1 - bs.Length
For b = 0 To bs.Length - 1
If bs(b) <> Data(i + b) Then Exit
Next
If b = bs.Length Then Return i
Next
Return -1
End Sub
The data is copied to a large array. Once a frame is found it is parsed and the array is trimmed using ByteConverter.ArrayCopy.
It is compatible with B4i, B4A and B4J.
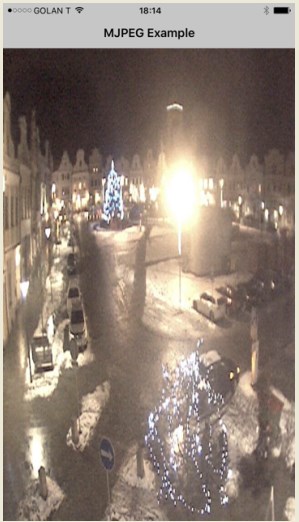
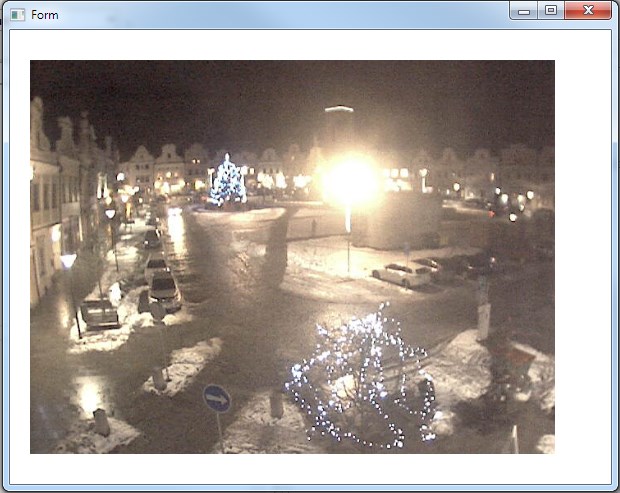
It works better in release mode.
You will need to handle disconnections.
Attachments
Last edited: