HI, All
I know nothing about JS.
A web-page is so:
No visible HTML-buttons with href-links to be catched.
Buttons are in DOM:
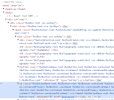
_OverrideUrl and _ShouldInterceptRequest events are not fired if to click.
Is it possible to catch clicking such buttons in WebView?
I know nothing about JS.
A web-page is so:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Page title</title>
<!-- Styles -->
<link href="https://domain/app.css" rel="stylesheet">
</head>
<body>
<!-- React root DOM -->
<div id="user">
</div>
<!-- React JS -->
<script src="https://domain/js/app.js" defer></script>
</body>
</html>
No visible HTML-buttons with href-links to be catched.
Buttons are in DOM:
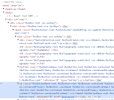
_OverrideUrl and _ShouldInterceptRequest events are not fired if to click.
Is it possible to catch clicking such buttons in WebView?