Attached is a small program of a bouncing (and rotating) smiley.
As you can see in the image the background is not just a solid color.
The code for this program:
You are more than welcome to try to run it on your android device. 
As you can see in the image the background is not just a solid color.
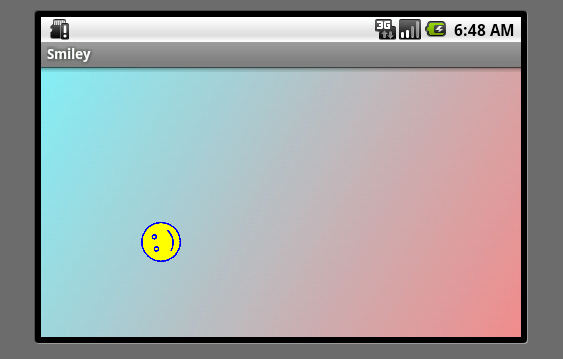
The code for this program:
B4X:
'Activity module
Sub Process_Globals
Dim x, y, vx, vy As Int
Dim boxSize As Int
Dim Rect As Rect
Dim timer1 As Timer
Dim SmileyBitmap As Bitmap
Dim Degrees As Int
End Sub
Sub Globals
Dim Canvas1 As Canvas
Dim Bitmap1 As Bitmap
End Sub
Sub Activity_Create(FirstTime As Boolean)
If FirstTime Then
Timer1.Initialize("Timer1", 30)
vx = 10dip 'smiley speed
vy = 10dip
x = 50%x 'smiley first position (middle of the screen
y = 50%y
boxSize = 40dip 'smiley size
Rect.Initialize(0, 0, 0, 0)
SmileyBitmap.Initialize(File.DirAssets, "smiley.gif") 'load the smiley file
End If
Activity.LoadLayout("smiley.bal") 'load the designer layout (just the gradient color in this case).
Canvas1.Initialize(Activity) 'Initialize the canvas (drawer) and makes it draw on the activity (form).
Bitmap1.Initialize3(Canvas1.Bitmap) 'Save a copy of the current background. This bitmap will be used to erase the smiley each time.
End Sub
Sub Activity_Pause
Timer1.Enabled = False
End Sub
Sub Activity_Resume
Timer1.Enabled = True
End Sub
Sub Timer1_Tick
If x + boxSize > 99%x Then
vx = -1 * Abs(vx)
Else If x < 1%x Then
vx = Abs(vx)
End If
If y + boxSize > 99%y Then
vy = -1 * Abs(vy)
Else If y < 1%y Then
vy = Abs(vy)
End If
Rect.Top = y
Rect.Left = x
Rect.Bottom = y + boxSize
Rect.Right = x + boxSize
Canvas1.DrawBitmap(Bitmap1, Rect, Rect) 'Erase the previous smiley
Activity.Invalidate2(Rect)
x = x + vx
y = y + vy
Rect.Top = y
Rect.Left = x
Rect.Bottom = y + boxSize
Rect.Right = x + boxSize
Degrees = (Degrees + 10) Mod 360
Canvas1.DrawBitmapRotated(SmileyBitmap, Null, Rect, Degrees) 'Draw the new smiley
Activity.Invalidate2(Rect)
End Sub
Sub Activity_Touch(Action As Int, tx As Float, ty As Float)
If Not(Action = Activity.ACTION_DOWN) Then Return
If tx > x - boxSize AND tx < x + boxSize AND _
ty > y - boxSize AND ty < y + boxSize Then
vx = -vx 'change the smiley direction if the user pressed on it
vy = -vy
End If
Canvas1.DrawCircle(tx, ty, 5dip, Colors.Red, False, 2dip)
Activity.Invalidate3(tx - 7dip, ty - 7dip, tx + 7dip, ty + 7dip)
End Sub