HttpUtils2 was replaced with OkHttpUtils2: https://www.b4x.com/android/forum/threads/okhttp-replaces-the-http-library.54723/
Both libraries are included in the IDE.
HttpUtils2 is a small framework that helps with communicating with web services (Http servers).
HttpUtils2 is an improved version of HttpUtils.
The advantages of HttpUtils2 over HttpUtils are:
HttpUtils2 requires Basic4android v2.00 or above.
It is made of two code modules: HttpUtils2Service and HttpJob (class module).
The two code modules are included in HttpUtils2 (attached project).
It depends on the following libraries: Http and StringUtils
How to use
- Dim a HttpJob object
- Initialize the Job and set the module that will handle the JobDone event.
The JobDone event is raised when a job completes.
The module can be an Activity, Service or class instance. You can use the Me keyword to reference the current module.
Note that CallSubDelayed is used to call the event.
- Call one of the following methods:
Download, Download2, PostString, PostBytes or PostFile. See HttpJob comments for more information.
- Handle the JobDone event and call Job.Release when done.
Note that the completion order may be different than the submission order.
To send credentials you should set Job.UserName and Job.Password fields before sending the request.
For example the following code sends three request. Two of the responses will be printed to the logs and the third will be set as the activity background:
This example and an example of downloading several images from Flickr are attached:
Starting from B4A v2.70, HttpUtils2 is included as a library in the IDE.
Relevant links
ImageDownloader - Service that makes it simple to efficiently download multiple images. Note that a simpler "FlickrViewer example" is available there.
DownloadService - Download files of any size with DownloadService. Includes progress monitoring and the ability to cancel a download.
Both libraries are included in the IDE.
HttpUtils2 is a small framework that helps with communicating with web services (Http servers).
HttpUtils2 is an improved version of HttpUtils.
The advantages of HttpUtils2 over HttpUtils are:
- Any number of jobs can run at the same time (each job is made of a single task)
- Simpler to use
- Simpler to modify
- Supports credentials
- GetString2 for encodings other than UTF8
- Download2 encodes illegal parameters characters (like spaces)
HttpUtils2 requires Basic4android v2.00 or above.
It is made of two code modules: HttpUtils2Service and HttpJob (class module).
The two code modules are included in HttpUtils2 (attached project).
It depends on the following libraries: Http and StringUtils
How to use
- Dim a HttpJob object
- Initialize the Job and set the module that will handle the JobDone event.
The JobDone event is raised when a job completes.
The module can be an Activity, Service or class instance. You can use the Me keyword to reference the current module.
Note that CallSubDelayed is used to call the event.
- Call one of the following methods:
Download, Download2, PostString, PostBytes or PostFile. See HttpJob comments for more information.
- Handle the JobDone event and call Job.Release when done.
Note that the completion order may be different than the submission order.
To send credentials you should set Job.UserName and Job.Password fields before sending the request.
For example the following code sends three request. Two of the responses will be printed to the logs and the third will be set as the activity background:
B4X:
Sub Activity_Create(FirstTime As Boolean)
Dim job1, job2, job3 As HttpJob
job1.Initialize("Job1", Me)
'Send a GET request
job1.Download2("http://www.b4x.com/print.php", _
Array As String("first key", "first value :)", "second key", "value 2"))
'Send a POST request
job2.Initialize("Job2", Me)
job2.PostString("http://www.b4x.com/print.php", "first key=first value&key2=value2")
'Send a GET request
job3.Initialize("Job3", Me)
job3.Download("http://www.b4x.com/forum/images/categories/android.png")
End Sub
Sub JobDone (Job As HttpJob)
Log("JobName = " & Job.JobName & ", Success = " & Job.Success)
If Job.Success = True Then
Select Job.JobName
Case "Job1", "Job2"
'print the result to the logs
Log(Job.GetString)
Case "Job3"
'show the downloaded image
Activity.SetBackgroundImage(Job.GetBitmap)
End Select
Else
Log("Error: " & Job.ErrorMessage)
ToastMessageShow("Error: " & Job.ErrorMessage, True)
End If
Job.Release
End Sub
This example and an example of downloading several images from Flickr are attached:
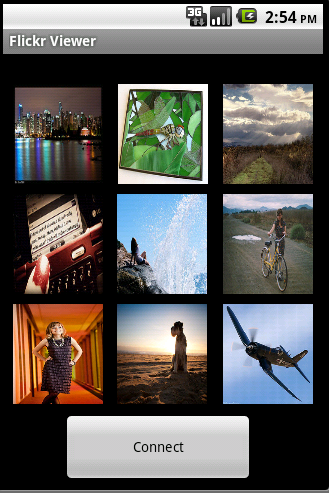
Starting from B4A v2.70, HttpUtils2 is included as a library in the IDE.
Relevant links
ImageDownloader - Service that makes it simple to efficiently download multiple images. Note that a simpler "FlickrViewer example" is available there.
DownloadService - Download files of any size with DownloadService. Includes progress monitoring and the ability to cancel a download.
Attachments
Last edited: