V1.5
Added: SetSkew() - To skew to image
Added: Reset() - Resets general image parameters (skew, position, rotation, handle, animation, flipped state)
I have put together a library designed for the purpose of displaying 2D images using OpenGL ES. The library needs Andrew Graham's libraries:
OpenGL ES library.
Reflection Library
Also, if you want to employ error checking you will also need Andrew's Threading library.
VIDEO: Jelly Beans
Key Features for the 2D Images
=======================================
* Pixel-Perfect image drawing (with unfiltered or filtered render options)
* Resize, rotate, flip, skew, change colour, alpha, blending modes, cropped areas - All faster than stock bitmap/canvas drawing
* Collision checks (only basic box-box checks)
* Anchor points - Each image has its own anchor hotspot (called handle in the library) so that the image can be rotated/flipped around a particular point
* An image can share the texture from an existing image (to save space and improve memory performance)
* Images don't need to be a power of 2 since the library will re-size them if required.
* Animation - Images can be animated easily by setting 'frames'. Image strips as well as image grids are supported
* Set specific areas of the image to draw
Key Features for the Display
=======================================
* Virtual display setup makes it easy to create a common display which scales to the devices physical screen size
* Global positioning (anchor/handle), scaling, and rotation which impacts all images.
* GL surface view can be made transparent so that GL images appear on top of regular views.
Have a play with the examples to see how the basic implementation works.
Hopefully the commands make sense.
OpenGL ES 2D Image Library v1.5 - Download link -- Select File then Download
NOTE: Demos should be run in Release Mode
V1.1
Added: Transparency for GL surface view. New subs = SetClsAlpha(), SetClsColor()
Changed: ClearScreen() to Cls()
V1.2
Added: Virtual display commands/functions
Added: SetRect() for images
V1.3
Added: GetLeft(), GetRight(), GetTop(), GetBottom(), AtPoint()
Added: More demos
V1.4a Minor change: Removed glBlendEquation
V1.4
Added: SetBlend - To change the rendering appearance of images (Alpha, Light,Dark,Mask)
Added: Jelly Beans demo
Changed: The way images are loaded. Instead of checking for surface changes every time Draw() is called, a dedicated (one-shot) LoadImages() sub is called instead.
Added: SetSkew() - To skew to image
Added: Reset() - Resets general image parameters (skew, position, rotation, handle, animation, flipped state)
I have put together a library designed for the purpose of displaying 2D images using OpenGL ES. The library needs Andrew Graham's libraries:
OpenGL ES library.
Reflection Library
Also, if you want to employ error checking you will also need Andrew's Threading library.
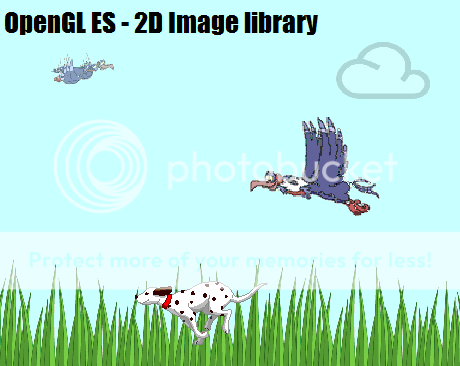
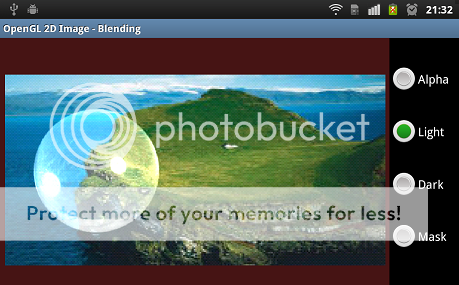
VIDEO: Jelly Beans
Key Features for the 2D Images
=======================================
* Pixel-Perfect image drawing (with unfiltered or filtered render options)
* Resize, rotate, flip, skew, change colour, alpha, blending modes, cropped areas - All faster than stock bitmap/canvas drawing
* Collision checks (only basic box-box checks)
* Anchor points - Each image has its own anchor hotspot (called handle in the library) so that the image can be rotated/flipped around a particular point
* An image can share the texture from an existing image (to save space and improve memory performance)
* Images don't need to be a power of 2 since the library will re-size them if required.
* Animation - Images can be animated easily by setting 'frames'. Image strips as well as image grids are supported
* Set specific areas of the image to draw
Key Features for the Display
=======================================
* Virtual display setup makes it easy to create a common display which scales to the devices physical screen size
* Global positioning (anchor/handle), scaling, and rotation which impacts all images.
* GL surface view can be made transparent so that GL images appear on top of regular views.
Have a play with the examples to see how the basic implementation works.
Hopefully the commands make sense.
OpenGL ES 2D Image Library v1.5 - Download link -- Select File then Download
NOTE: Demos should be run in Release Mode
V1.1
Added: Transparency for GL surface view. New subs = SetClsAlpha(), SetClsColor()
Changed: ClearScreen() to Cls()
V1.2
Added: Virtual display commands/functions
Added: SetRect() for images
V1.3
Added: GetLeft(), GetRight(), GetTop(), GetBottom(), AtPoint()
Added: More demos
V1.4a Minor change: Removed glBlendEquation
V1.4
Added: SetBlend - To change the rendering appearance of images (Alpha, Light,Dark,Mask)
Added: Jelly Beans demo
Changed: The way images are loaded. Instead of checking for surface changes every time Draw() is called, a dedicated (one-shot) LoadImages() sub is called instead.
Last edited: