This B4R example/library works with DHT11 and DHT22 temperature and humidity sensors:
This inexpensive device (DHT11) allows for measure temperature and humidity:
Attached an example that reads DHT11 sensor (on Arduino pin 4) and DHT22 sensor (on pin 3) at same time
In attached file (rdht.zip), you'll find the B4R library and the Arduino lib (dhtlib.zip)
DHT is not a lib included on Arduino Skecth and it seems there're two ways to get working a library not included in the Arduino default libraries:
1 Option) Download the zip from https://github.com/adafruit/DHT-sensor-library and install on Arduino IDE with Sketch Menu --> Include Library --> Add Zip library or Sketch Menu --> Include Library --> Manage Library and do a Search for it. After, descompress the rDHT.zip on B4R Libraries Folder
2 Option) Modifying the rDht.h and rDht.xml for search the Arduino library (Dht.h and Dht.h) on B4R folder (it won't work on Arduino IDE) --> See posts #8-#13
For now, I'm more comfortable with first option as it permits to test the Arduino with several ino programs before to testing on B4R, but the choice it's yours.
rDhtlib v2.00:
- Included DHT11 and DHT22 sensors (decompress to B4R Libraries folder)
This inexpensive device (DHT11) allows for measure temperature and humidity:
- Very Low cost
- 3 to 5V power and I/O
- 2.5mA max current use during conversion (while requesting data)
- Good for 20-80% humidity readings with 5% accuracy
- Good for 0-50°C temperature readings ±2°C accuracy
- No more than 1 Hz sampling rate (once every second)
- Body size 15.5mm x 12mm x 5.5mm
- 4 pins with 0.1" spacing
- Low cost
- 3 to 5V power and I/O
- 2.5mA max current use during conversion (while requesting data)
- Good for 0-100% humidity readings with 2-5% accuracy
- Good for -40 to 125°C temperature readings ±0.5°C accuracy
- No more than 0.5 Hz sampling rate (once every 2 seconds)
- Body size 15.1mm x 25mm x 7.7mm
- 4 pins with 0.1" spacing
DHT11 & DHT22
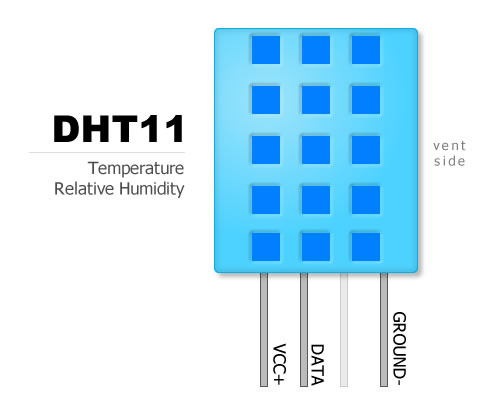
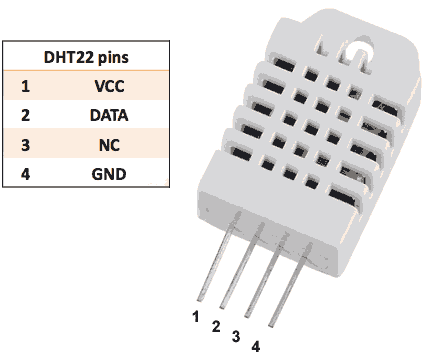
DHT11 & DHT22
Attached an example that reads DHT11 sensor (on Arduino pin 4) and DHT22 sensor (on pin 3) at same time
B4X:
Sub Process_Globals
'These global variables will be declared once when the application starts.
'Public variables can be accessed from all modules.
Public Serial1 As Serial
Public DHT11sensor As dht 'DHT11 sensor
Public DHT22sensor As dht 'DHT22 sensor
Public Timer1 As Timer 'Timer for reading DHT11 measures at desired interval
Public DHT11pin,DHT22pin As Pin 'Arduino Pin connected to DHT11 signal
Public Interval As Int 'Interval as seconds
Dim humidity,temperature As Double 'Humidity/Temperature DHT11 readings
End Sub
Private Sub AppStart
Serial1.Initialize(115200)
Log("AppStart")
Interval=2 '2 sec Timer Interval (for DHT22 measures)
DHT11pin.Initialize(4,DHT11pin.MODE_INPUT) 'Initialize at Arduino Pin 4
DHT22pin.Initialize(3,DHT22pin.MODE_INPUT) 'Initialize at Arduino Pin 3
Timer1.Initialize("Timer1_Tick",Interval*1000)
Timer1.Enabled=True 'Begin Timer
End Sub
Sub Timer1_Tick
DHT11sensor.Read11(DHT11pin.PinNumber) 'Reading the DHT11 measure
humidity=DHT11sensor.GetHumidity 'Get humidity from readed measure
temperature=DHT11sensor.GetTemperature 'Get temperature from readed measure
Log("DHT11 ","Humidity = ",humidity, " %", " Temperature =",temperature, " Cº")
Delay(200)
DHT22sensor.Read22(DHT22pin.PinNumber) 'Reading the DHT22 measure
humidity=DHT22sensor.GetHumidity 'Get humidity from readed measure
temperature=DHT22sensor.GetTemperature 'Get temperature from readed measure
Log("DHT22 ","Humidity = ",NumberFormat(humidity,2,1), " %", " Temperature =",NumberFormat(temperature,2,1), " Cº")
End Sub
In attached file (rdht.zip), you'll find the B4R library and the Arduino lib (dhtlib.zip)
DHT is not a lib included on Arduino Skecth and it seems there're two ways to get working a library not included in the Arduino default libraries:
1 Option) Download the zip from https://github.com/adafruit/DHT-sensor-library and install on Arduino IDE with Sketch Menu --> Include Library --> Add Zip library or Sketch Menu --> Include Library --> Manage Library and do a Search for it. After, descompress the rDHT.zip on B4R Libraries Folder
2 Option) Modifying the rDht.h and rDht.xml for search the Arduino library (Dht.h and Dht.h) on B4R folder (it won't work on Arduino IDE) --> See posts #8-#13
For now, I'm more comfortable with first option as it permits to test the Arduino with several ino programs before to testing on B4R, but the choice it's yours.
rDhtlib v2.00:
- Included DHT11 and DHT22 sensors (decompress to B4R Libraries folder)
Attachments
Last edited: