This is a simple example of a traffic light implementation using Raspberry Pi 2.
The red, yellow and green leds are connected to pins 27, 28 and 29 (see the numbering scheme: http://pi4j.com/pins/model-b-plus.html).
Using a timer we switch between four different states:
The complete code:
It depends on jPi4J v1.5+: https://www.b4x.com/android/forum/threads/jpi4j-raspberry-pi-gpio-controller.37493
The red, yellow and green leds are connected to pins 27, 28 and 29 (see the numbering scheme: http://pi4j.com/pins/model-b-plus.html).
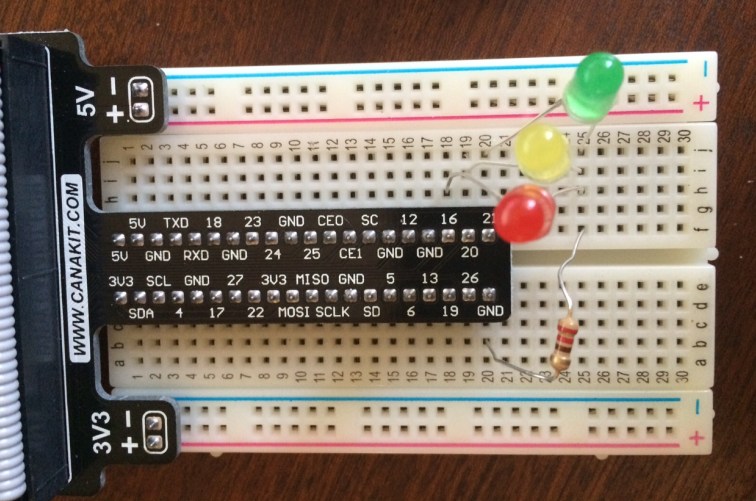
Using a timer we switch between four different states:
The complete code:
B4X:
Sub Process_Globals
Type State (Duration As Int, Red As Boolean, Yellow As Boolean, Green As Boolean)
Private timer1 As Timer
Private controller As GpioController
Private pinRed, pinYellow, pinGreen As GpioPinDigitalOutput
Private states As List
Private currentStateIndex As Int
End Sub
Sub AppStart (Args() As String)
controller.Initialize
pinRed.Initialize(27, False)
pinYellow.Initialize(28, False)
pinGreen.Initialize(29, False)
states.Initialize
states.Add(CreateState(4000, True, False, False)) 'only red
states.Add(CreateState(2000, True, True, False)) 'red + yellow
states.Add(CreateState(4000, False, False, True)) 'only green
states.Add(CreateState(2000, False, True, False)) 'only yellow
timer1.Initialize("timer1", 1000)
timer1.Enabled = True
currentStateIndex = -1 'it will be incremented in Timer1_Tick
Timer1_Tick 'start with the first state
StartMessageLoop
End Sub
Private Sub Timer1_Tick
currentStateIndex = (currentStateIndex + 1) mod states.Size
Dim st As State = states.Get(currentStateIndex)
pinRed.State = st.Red
pinYellow.State = st.Yellow
pinGreen.State = st.Green
timer1.Interval = st.Duration
End Sub
Private Sub CreateState(Duration As Int, Red As Boolean, Yellow As Boolean, Green As Boolean) As State
Dim st As State
st.Initialize
st.Duration = Duration
st.Red = Red
st.Yellow = Yellow
st.Green = Green
Return st
End Sub
It depends on jPi4J v1.5+: https://www.b4x.com/android/forum/threads/jpi4j-raspberry-pi-gpio-controller.37493