Parse is a company that provides several services for mobile applications.
www.parse.com
This library is a simple wrapper for ParseObject and ParseQuery.
Using these objects it is simple to store and retrieve data on the cloud.
The web interface:
This library is considered a beta library. If there will be interest in this library and the other features provided by Parse.com then it will be further developed. Please provide your feedback.
All the operations which need to access their online service are done asynchronously and raise events when done.
Please read their guide for more information about the usage.
Installation instructions:
- In order to use this library you should sign to Parse.com and create a new application.
You will get two keys: ClientKey and ApplicationId. Both are required.
- You also need to download their SDK. You will find a jar file named Parse-0.3.8.jar.
You should rename it to ParseNative.jar and copy it to the libraries folder.
- Download the attached zip file and copy all three files to the libraries folder.
- You should add a reference to Parse and ParseNative in the libs tab.
A simple program that creates several objects and then retrieves those objects:
www.parse.com
This library is a simple wrapper for ParseObject and ParseQuery.
Using these objects it is simple to store and retrieve data on the cloud.
The web interface:
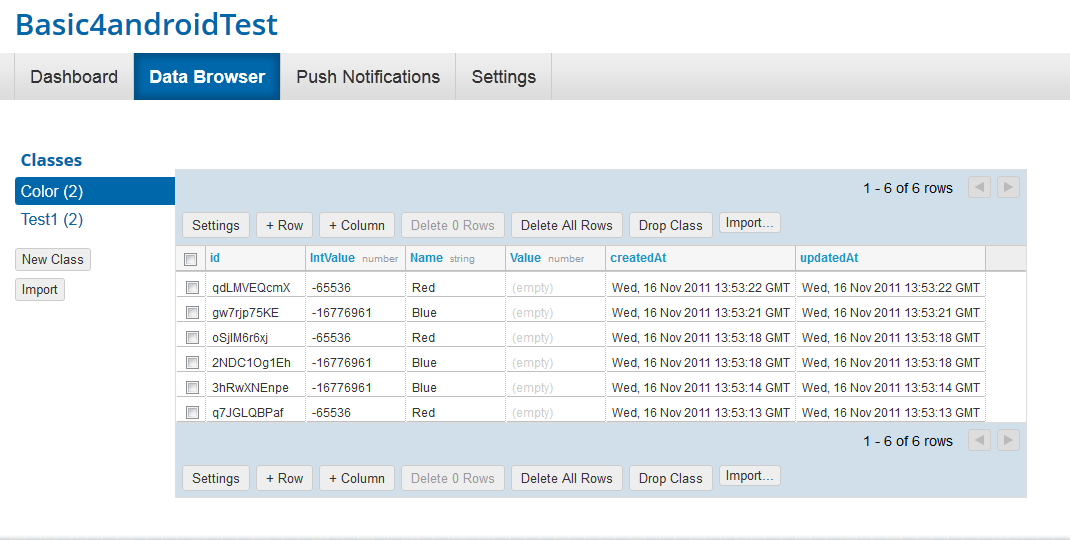
This library is considered a beta library. If there will be interest in this library and the other features provided by Parse.com then it will be further developed. Please provide your feedback.
All the operations which need to access their online service are done asynchronously and raise events when done.
Please read their guide for more information about the usage.
Installation instructions:
- In order to use this library you should sign to Parse.com and create a new application.
You will get two keys: ClientKey and ApplicationId. Both are required.
- You also need to download their SDK. You will find a jar file named Parse-0.3.8.jar.
You should rename it to ParseNative.jar and copy it to the libraries folder.
- Download the attached zip file and copy all three files to the libraries folder.
- You should add a reference to Parse and ParseNative in the libs tab.
A simple program that creates several objects and then retrieves those objects:
B4X:
Sub Process_Globals
Dim AppId, ClientKey As String
AppId = "YourAppId"
ClientKey = "YoudClientKey"
Dim Parse As Parse
End Sub
Sub Globals
End Sub
Sub Activity_Create(FirstTime As Boolean)
If FirstTime Then
Parse.Initialize(AppId, ClientKey)
End If
Activity.AddMenuItem("Add Items", "AddItems")
Activity.AddMenuItem("List Items", "ListItems")
Activity.AddMenuItem("Delete Items", "DeleteItems")
End Sub
Sub AddItems_Click
Dim po As ParseObject
po.Initialize("Color")
po.Put("Name", "Red")
po.Put("IntValue", Colors.Red)
po.Save("po")
Dim po As ParseObject
po.Initialize("Color")
po.Put("Name", "Blue")
po.Put("IntValue", Colors.Blue)
po.Save("po")
ProgressDialogShow("Waiting for response.")
End Sub
Sub po_DoneSave (Success As Boolean)
ProgressDialogHide
Dim po As ParseObject
po = Sender
Dim msg As String
msg = "Saving Color: " & po.GetString("Name") & " Success=" & Success
Log(msg)
ToastMessageShow(msg, False)
If Success = False Then
Log(LastException.Message)
End If
End Sub
Sub ListItems_Click
Dim query As ParseQuery
query.Initialize("Color")
query.OrderBy("Name", True)
query.Find("query", 1)
ProgressDialogShow("Waiting for response.")
End Sub
Sub query_DoneFind (Success As Boolean, ListOfPO As List, TaskId As Int)
ProgressDialogHide
If Success = False Then
Log("Error: " & LastException.Message)
ToastMessageShow("Error: " & LastException.Message, True)
Else
Dim sb As StringBuilder
sb.Initialize
For i = 0 To ListOfPO.Size - 1
Dim po As ParseObject
po = ListOfPO.Get(i)
sb.Append(po.GetString("Name")).Append("=").Append(po.GetInt("IntValue")).Append(CRLF)
Next
Msgbox(sb.ToString, "")
End If
End Sub
Sub DeleteItems_Click
Dim query As ParseQuery
query.Initialize("Color")
query.Find("DeleteItems", 1)
ProgressDialogShow("Waiting for response.")
End Sub
Sub DeleteItems_DoneFind (Success As Boolean, ListOfPO As List, TaskId As Int)
ProgressDialogHide
If Success = False Then
Log("Error: " & LastException.Message)
ToastMessageShow("Error: " & LastException.Message, True)
Else
For i = 0 To ListOfPO.Size - 1
Dim po As ParseObject
po = ListOfPO.Get(i)
po.Delete("delete")
ProgressDialogShow("Waiting for response.")
Next
End If
End Sub
Sub Delete_DoneDelete (Success As Boolean)
ProgressDialogHide
Dim po As ParseObject
po = Sender
Log(po.ObjectId & " delete, success = " & Success)
End Sub
Sub Activity_Pause(UserClosed As Boolean)
End Sub
Sub Activity_Resume
End Sub