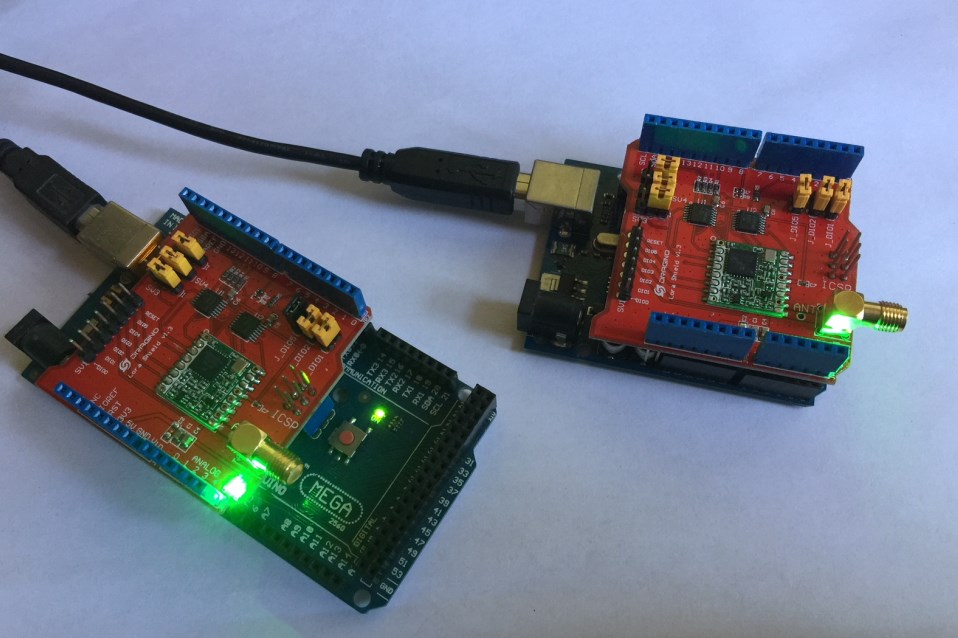
This is a wrapper for RadioHead library. Currently only RF95 is exposed.
RadioHead documentation: http://www.airspayce.com/mikem/arduino/RadioHead/classRH__RF95.html#details
"Driver to send and receive unaddressed, unreliable datagrams via a LoRa capable radio transceiver."
Installation steps:
1. Download the attached library and copy to the additional libraries folder.
2. Download RadioHead and copy it to Arduino libraries folder (by default it is Documents\Arduino\libraries).
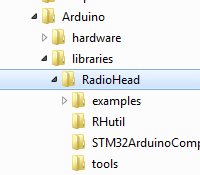
Usage:
1. Initialize RF95 and make sure that it returns True.
2. Set the frequency.
2. Send messages with RF95.Send.
3. Handle the DataAvailable event.
Max message length is 251 bytes.
Example:
Program A:
B4X:
Sub Process_Globals
Public Serial1 As Serial
Private rf95 As RF95
Private ser As B4RSerializator
End Sub
Private Sub AppStart
Serial1.Initialize(115200)
Log("AppStart")
If rf95.Initialize("rf95_DataAvailable") = False Then
Log("Failed to init!!!")
Return
End If
rf95.Frequency = 868.1
End Sub
Sub rf95_DataAvailable (Data() As Byte)
Dim objects(5) As Object
Dim res() As Object = ser.ConvertBytesToArray(Data, objects)
If res.Length > 0 Then
Log("Message received from: ", res(0))
Dim time As ULong = res(1)
Log("Message time: ", time)
rf95.Send(ser.ConvertArrayToBytes(Array("Mega - Thank you!", Millis)))
End If
End Sub
Program B:
B4X:
Sub Process_Globals
Public Serial1 As Serial
Private rf95 As RF95
Private timer1 As Timer
Private ser As B4RSerializator
End Sub
Private Sub AppStart
Serial1.Initialize(115200)
Log("AppStart")
If rf95.Initialize("rf95_DataAvailable") = False Then
Log("Failed to init!!!")
Return
End If
rf95.Frequency = 868.1
timer1.Initialize("timer1_Tick", 1000)
timer1.Enabled = True
End Sub
Sub Timer1_Tick
Dim b() As Byte = ser.ConvertArrayToBytes(Array("Arduino Uno", Millis))
If rf95.Send(b) = False Then
Log("Failed to send message")
End If
End Sub
Sub rf95_DataAvailable (Data() As Byte)
Dim objects(5) As Object
Dim res() As Object = ser.ConvertBytesToArray(Data, objects)
If res.Length > 0 Then
Log("Message received from: ", res(0))
Dim time As ULong = res(1)
Log("Message time: ", time)
End If
End Sub
Examples depend on rRadioHead and rRandomAccessFile libraries.