If you are using B4A v5.80+ then please follow this tutorial: https://www.b4x.com/android/forum/threads/google-maps.63930/#post-404386
GoogleMaps library requires v2.50 or above.
GoogleMaps library allows you to add Google maps to your application. This library requires Android 3+ and will only work on devices with Google Play service.
This tutorial will cover the configuration steps required for showing a map.
1. Download Google Play services - From the IDE choose Run AVD Manager and then choose Tools - SDK Manager. Select Google Play services under the Extras node and install it:
2. Copy google-play-services.jar to the libraries folder - This file is available under:
2.5. Download the attached library, unzip it and copy to the libraries folder.
3. Find the key signature - Your application must be signed with a private key other than the debug key. After you select a new or existing key file (Tools - Private Sign Key) you should reopen the private key dialog. The signature information will be displayed (increase the dialog size as needed).
The value after SHA1 is required for the next step:
4. Create an API project - Follow these steps and create an API project.
You should follow the steps under "Creating an API Project" and "Obtaining an API key".
Tips:
- Make sure to select "Google Maps Android API v2" in the services list and not one of the other similar services.
- Under "Simple API Access" you should select "Key for Android apps (with certificates".
5. Add the following code to the manifest editor:
You should replace the value after android:value with the key you received in the previous step.
6. Add an #AdditionalRes attribute to the main activity:
You should add a reference to Google play resources by adding the following line:
For example:
#AdditionalRes: C:\android-sdk-windows\extras\google\google_play_services\libproject\google-play-services_lib\res, com.google.android.gms
Run the following code:
You should see:
If you see a "white map" then there is most probably a mismatch between the: package name, sign key and the API key (from the manifest editor).
Google documentation: https://developers.google.com/maps/documentation/android/intro
Note that there is a required attribution which you must include in your app (see above link). You can get the string by calling MapFragment.GetOpenSourceSoftwareLicenseInfo.
V1.01: Fixes a bug in AddMarker2.
GoogleMaps library requires v2.50 or above.
GoogleMaps library allows you to add Google maps to your application. This library requires Android 3+ and will only work on devices with Google Play service.
This tutorial will cover the configuration steps required for showing a map.
1. Download Google Play services - From the IDE choose Run AVD Manager and then choose Tools - SDK Manager. Select Google Play services under the Extras node and install it:
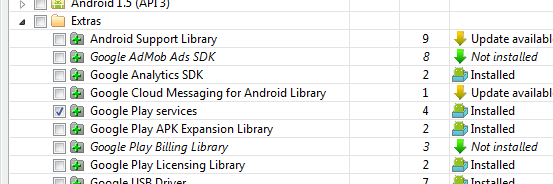
2. Copy google-play-services.jar to the libraries folder - This file is available under:
You should copy it to the libraries folder.C:\<android sdk>\extras\google\google_play_services\libproject\google-play-services_lib\libs (ignore the extra space that the forum script insists on adding)
2.5. Download the attached library, unzip it and copy to the libraries folder.
3. Find the key signature - Your application must be signed with a private key other than the debug key. After you select a new or existing key file (Tools - Private Sign Key) you should reopen the private key dialog. The signature information will be displayed (increase the dialog size as needed).
The value after SHA1 is required for the next step:
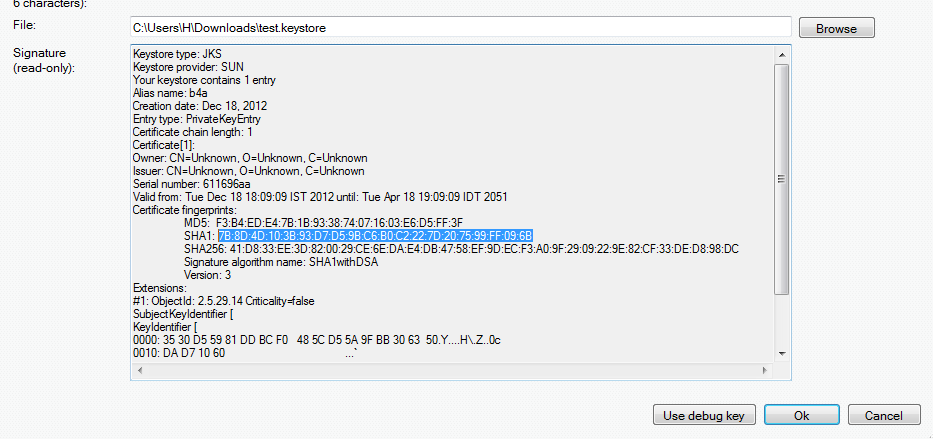
4. Create an API project - Follow these steps and create an API project.
You should follow the steps under "Creating an API Project" and "Obtaining an API key".
Tips:
- Make sure to select "Google Maps Android API v2" in the services list and not one of the other similar services.
- Under "Simple API Access" you should select "Key for Android apps (with certificates".
5. Add the following code to the manifest editor:
B4X:
AddManifestText( <permission
android:name="$PACKAGE$.permission.MAPS_RECEIVE"
android:protectionLevel="signature"/>
<uses-feature android:glEsVersion="0x00020000" android:required="true"/>)
AddApplicationText(<meta-data
android:name="com.google.android.maps.v2.API_KEY"
android:value="AIzaSyCzspmxxxxxxxxxxxxx"/>
<meta-data android:name="com.google.android.gms.version"
android:value="@integer/google_play_services_version"
/>)
AddPermission(android.permission.ACCESS_NETWORK_STATE)
6. Add an #AdditionalRes attribute to the main activity:
You should add a reference to Google play resources by adding the following line:
B4X:
#AdditionalRes: <google-play-services res folder>, com.google.android.gms
#AdditionalRes: C:\android-sdk-windows\extras\google\google_play_services\libproject\google-play-services_lib\res, com.google.android.gms
Run the following code:
B4X:
'Activity module
Sub Process_Globals
End Sub
Sub Globals
Dim mFragment As MapFragment
Dim gmap As GoogleMap
Dim MapPanel As Panel
End Sub
Sub Activity_Create(FirstTime As Boolean)
MapPanel.Initialize("")
Activity.AddView(MapPanel, 0, 0, 100%x, 100%y)
If mFragment.IsGooglePlayServicesAvailable = False Then
ToastMessageShow("Google Play services not available.", True)
Else
mFragment.Initialize("Map", MapPanel)
End If
End Sub
Sub Map_Ready
Log("map ready")
gmap = mFragment.GetMap
If gmap.IsInitialized = False Then
ToastMessageShow("Error initializing map.", True)
Else
gmap.AddMarker(36, 15, "Hello!!!")
Dim cp As CameraPosition
cp.Initialize(36, 15, gmap.CameraPosition.Zoom)
gmap.AnimateCamera(cp)
End If
End Sub
You should see:
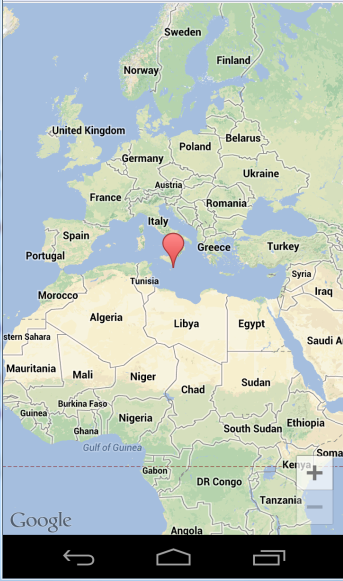
If you see a "white map" then there is most probably a mismatch between the: package name, sign key and the API key (from the manifest editor).
Google documentation: https://developers.google.com/maps/documentation/android/intro
Note that there is a required attribution which you must include in your app (see above link). You can get the string by calling MapFragment.GetOpenSourceSoftwareLicenseInfo.
V1.01: Fixes a bug in AddMarker2.
Attachments
Last edited: