AsyncStreamsObject is a small framework built over AsyncStreams.
Instead of sending and receiving bytes, AsyncStreamsObject allows you to send and receive objects. This makes it much simpler to communicate with other devices.
AsyncStreamsObject uses the WriteStream method of AsyncStreams. This method was introduced in RandomAccessFile v1.5.
You can use AsyncStreamsObject when you implement both sides of the connection.
Supported objects
WriteObject supports: Lists, Arrays, Maps, Strings, primitive types and user defined types.
WriteBitmap allows you to send bitmaps.
WriteFile allows you to send any file.
All the three write methods expect a key (string) and a value (the actual object).
The NewObject event is raised when an object is received. You should use the key to decide how to handle the object. This code is taken from the attached example:
The ObjectSent event is raised after a value was successfully sent.
Initializing AsyncStreamsObject
Two steps are required. First you should call AsyncStreamsObject.Initialize and pass the target module and event name:
Once there is a connection you should call AsyncStreamsObject.Start:
Note that you can use any type of connection that provides an input stream and output stream.
The Terminated event is raised when the connection is closed.
The attached example connects two devices over the local network. You need to set the IP address of one of the devices and press on the Connect button. Once connect you can send data between the devices.
AsyncStreamsObject class is included in the attached example.
Instead of sending and receiving bytes, AsyncStreamsObject allows you to send and receive objects. This makes it much simpler to communicate with other devices.
AsyncStreamsObject uses the WriteStream method of AsyncStreams. This method was introduced in RandomAccessFile v1.5.
You can use AsyncStreamsObject when you implement both sides of the connection.
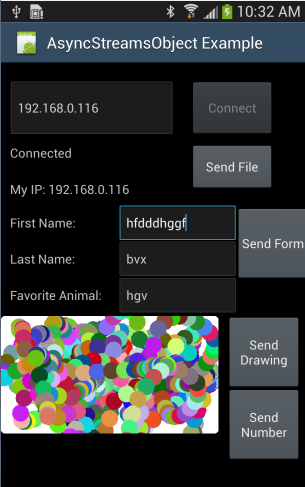
Supported objects
WriteObject supports: Lists, Arrays, Maps, Strings, primitive types and user defined types.
WriteBitmap allows you to send bitmaps.
WriteFile allows you to send any file.
All the three write methods expect a key (string) and a value (the actual object).
The NewObject event is raised when an object is received. You should use the key to decide how to handle the object. This code is taken from the attached example:
B4X:
Sub astreamO_NewObject(Key As String, Value As Object)
Select Key
Case "form"
Dim m As Map = Value 'Value is a Map
txtFirst.Text = m.Get("first")
txtLast.Text = m.Get("last")
txtAnimal.Text = m.Get("animal")
Case "simple value"
Dim number As Int = Value
ToastMessageShow("Received lucky number: " & number, False)
Case "image"
Dim bmp As Bitmap = Value
Dim r As Rect
r.Initialize(0, 0, Panel1.Width, Panel1.Height)
cvs.DrawBitmap(bmp, Null, r)
Panel1.Invalidate
Case "file"
Dim fileName As String = value
Log("Received file. File size: " & File.Size(astreamO.TempFolder, fileName))
End Select
End Sub
The ObjectSent event is raised after a value was successfully sent.
B4X:
Sub astreamO_ObjectSent (Key As String)
Log("Object sent: " & Key)
End Sub
Initializing AsyncStreamsObject
Two steps are required. First you should call AsyncStreamsObject.Initialize and pass the target module and event name:
B4X:
astreamO.Initialize(Me, "astreamO")
Once there is a connection you should call AsyncStreamsObject.Start:
B4X:
Sub StartAstream(s As Socket)
astreamO.Start(s.InputStream, s.OutputStream)
SetUIState
End Sub
The Terminated event is raised when the connection is closed.
The attached example connects two devices over the local network. You need to set the IP address of one of the devices and press on the Connect button. Once connect you can send data between the devices.
AsyncStreamsObject class is included in the attached example.
Attachments
Last edited: