DOWNLOAD THE LATEST VERSION OF BANANO:
https://www.b4x.com/android/forum/threads/banano-progressive-web-app-library.99740/#post-627764
Check out part 1 of this tutorial here: https://www.b4x.com/android/forum/threads/banano-v2-b4j-abstract-designer-part-1.101531/#post-637557
INTRODUCTION
Now that we have our custom view created, let's use it. As said in part 1, there are a couple of ways which I will go in to deeper now.
But first, I give you the steps on how to create a library out of part 1. This will allow us to use Sub + TAB in the B4J IDE.
CREATING A LIBRARY
This is a two step process. Make sure you have the following lines in your library AppStart code:
Now for step 1, run your library in release mode. The .js, .dependson (.php if you use it) and _Files.zip (if you have assets in the Files tab) will be created in your Additional Libraries folder. It will transpile everything in each .bas file. The Main class will be skipped.
Step 2 is just compiling the library with the B4J IDE 'Compile To Library'
Give it the same name as you have used in the BANano.Initialize declaration. The .jar and .xml files will also be generated in your Additional Libraries folder.
So to summerize: you will now have the following files in your Additional Libraries folder:
1. USING THE CUSTOM VIEW IN A LAYOUT
Make a new B4J UI project, and the BAnano.jar and yourlib.jar in the libraries.
also, unzip the yourlib_Files.zip file to your projects Files folder (watch out that you do not make a subfolder!).
Again let's start with adding the default BANano project main code:
Save and open the Abstract Designer. In the Views Menu, under custom views you will find your newly created custom views:
Example:
So, now we add our Custom views. You will notice the Custom properties in the Properties Pane. Only the following properties can be used (the rest is ignored):
Once you are satisfied with your design, save it and generate the events you want to use:
This makes sense, as a single layout matches up with one single view. In case of an array of such layouts, the is no One-to-One relation. But we go into this deeper in just a second.
The code will be generated for you:
All you have to do now is load your newly created layout:
This is just normal B4J stuff!
Now you can code as you are used to.
2. ADDING CUSTOM VIEWS BY CODE
This is equally similar to normal B4J behaviour. First add a declaration of a View in globals:
Now initialize and add the button:
The rest is again just normal. Type Sub + TAB and you can insert the events of the SKButton for example:
3. LOADING A LAYOUT MULTIPLE TIMES
Sometimes, you are going to want to re-use a certain layout multiple times. This can for example be because you made a layout for a list item, and now want to re-use it for each item in your list.
This can be done using the BANano.LoadLayoutArray method:
Nothing difficult here. The final parameter in LoadLayoutArray() can be used to clear the parent on which you are loading the layout (in this case #r3).
The method does return a 'unique' number. This is very useful to get all the views from your layout. We do this with the BANano.GetAllViewsFromLayoutArray() method.
The GetAllViewsFromLayoutArray() method returns a map with all the Views in it, for that layout, with instance 'unique number'.
So you can just grab Views and start manipulating them.
There is also a 'helper' method BANano.GetSuffixFromID() to know what this 'unique' number is, in case for example you want to make changes further in your code in a certain event.
NOTE:
Now with the support of the B4X Sender keyword, this is even easier:
This concludes the 2 part tutorial of the new UI system in BAnano v2. Possibilities are endless and so much closer to standard B4J than the UI system v1. But, we learn of our mistakes
Alwaysbusy
https://www.b4x.com/android/forum/threads/banano-progressive-web-app-library.99740/#post-627764
Check out part 1 of this tutorial here: https://www.b4x.com/android/forum/threads/banano-v2-b4j-abstract-designer-part-1.101531/#post-637557
INTRODUCTION
Now that we have our custom view created, let's use it. As said in part 1, there are a couple of ways which I will go in to deeper now.
But first, I give you the steps on how to create a library out of part 1. This will allow us to use Sub + TAB in the B4J IDE.
CREATING A LIBRARY
This is a two step process. Make sure you have the following lines in your library AppStart code:
B4X:
' start the build
#if release
BANano.BuildAsLibrary()
#else
BANano.Build(File.DirApp)
#end if
Now for step 1, run your library in release mode. The .js, .dependson (.php if you use it) and _Files.zip (if you have assets in the Files tab) will be created in your Additional Libraries folder. It will transpile everything in each .bas file. The Main class will be skipped.
Step 2 is just compiling the library with the B4J IDE 'Compile To Library'
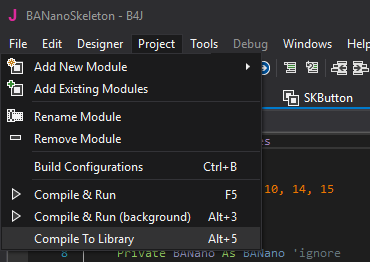
Give it the same name as you have used in the BANano.Initialize declaration. The .jar and .xml files will also be generated in your Additional Libraries folder.
So to summerize: you will now have the following files in your Additional Libraries folder:
B4X:
yourlib.js
yourlib.dependsOn
yourlib.jar
yourlib.xml
yourlib.php (if you have used inline php in your library)
yourlib_Files.zip (if you had assets in your Files tab)
1. USING THE CUSTOM VIEW IN A LAYOUT
Make a new B4J UI project, and the BAnano.jar and yourlib.jar in the libraries.
also, unzip the yourlib_Files.zip file to your projects Files folder (watch out that you do not make a subfolder!).
Again let's start with adding the default BANano project main code:
B4X:
#Region Project Attributes
#MainFormWidth: 600
#MainFormHeight: 600
#IgnoreWarnings: 16, 10, 14, 15
#End Region
Sub Process_Globals
Private BANano As BANano 'ignore
End Sub
Sub AppStart (Form1 As Form, Args() As String)
' you can change some output params here
BANano.Initialize("BANano", "BANanoSkeleton",12)
BANano.HTML_NAME = "index.html"
BANano.Header.Title="BANano Skeleton"
BANano.ExternalTestConnectionServer = "http://gorgeousapps.com"
BANano.Header.AddCSSFile("skeleton-all.min.css")
' start the build
BANano.Build(File.DirApp)
ExitApplication
End Sub
' HERE STARTS YOUR APP
Sub BANano_Ready()
End Sub
Save and open the Abstract Designer. In the Views Menu, under custom views you will find your newly created custom views:
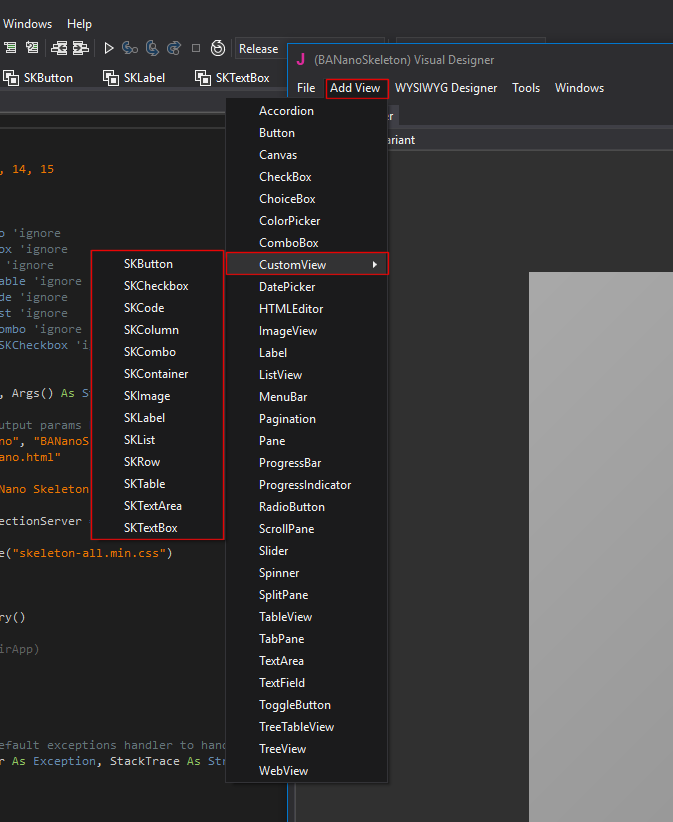
Before we start using our views, a quick note: as currently B4J Custom views can only set their parent to main, make sure there is some space between each view to allow BANanos own algorithm to determine who is the parent. The absolute position of a view in the Designer has no relevance in BANano anyway.
Example:
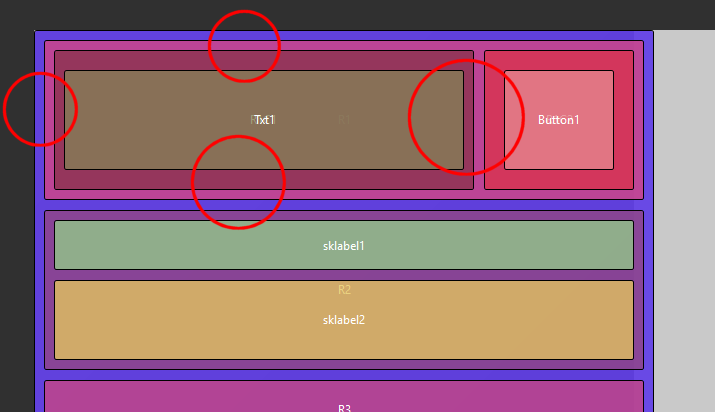
So, now we add our Custom views. You will notice the Custom properties in the Properties Pane. Only the following properties can be used (the rest is ignored):
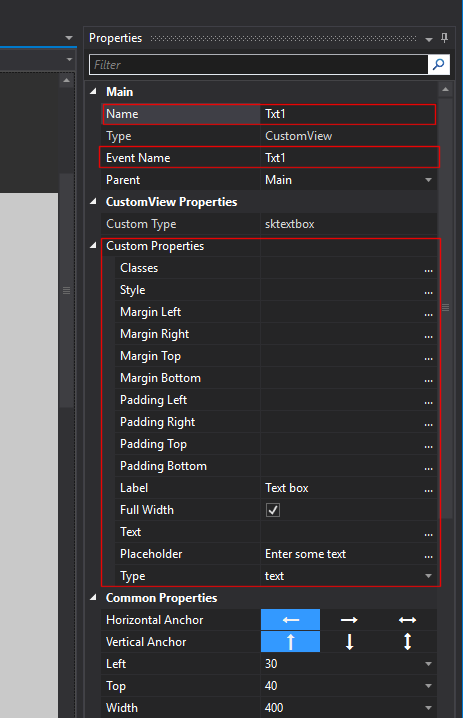
Once you are satisfied with your design, save it and generate the events you want to use:
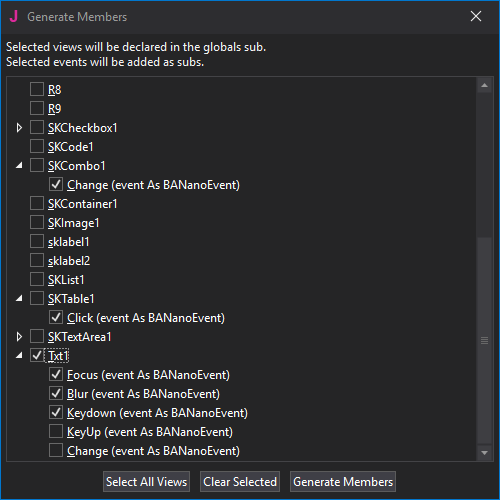
IMPORTANT: If you plan to load this layout only ONCE at the time with BANano.LoadLayout(), you can select the view itself (e.g. Txt1 in this example) to generate the Private Txt1 As SKTextBox line. If you plan to use BANano.LoadLayoutArray(), then you can NOT use this.
This makes sense, as a single layout matches up with one single view. In case of an array of such layouts, the is no One-to-One relation. But we go into this deeper in just a second.
The code will be generated for you:
B4X:
Sub Process_Globals
Private BANano As BANano
...
Private Txt1 As SKTextBox
End Sub
...
Sub Txt1_Focus (event As BANanoEvent)
End Sub
Sub Txt1_Blur (event As BANanoEvent)
End Sub
Sub Txt1_Keydown (event As BANanoEvent)
End Sub
Sub Txt1_KeyUp (event As BANanoEvent)
End Sub
Sub Txt1_Change (event As BANanoEvent)
End Sub
Sub Button1_Click (event As BANanoEvent)
End Sub
Sub SKTable1_Click (event As BANanoEvent)
End Sub
All you have to do now is load your newly created layout:
B4X:
' HERE STARTS YOUR APP
Sub BANano_Ready()
...
BANano.LoadLayout("#body", "layout1")
...
End Sub
This is just normal B4J stuff!
2. ADDING CUSTOM VIEWS BY CODE
This is equally similar to normal B4J behaviour. First add a declaration of a View in globals:
B4X:
Private btn As SKButton
Now initialize and add the button:
B4X:
' create a dynamic button, not located in the Layout
btn.Initialize(Me, "Button2", "Button2")
btn.Text = "Dynamic Button"
btn.AddToParent("R2")
IMPORTANT NOTE: The first param in Initialize() MUST be Me. Only the class where the View is added to will be able to handle the events.
The rest is again just normal. Type Sub + TAB and you can insert the events of the SKButton for example:
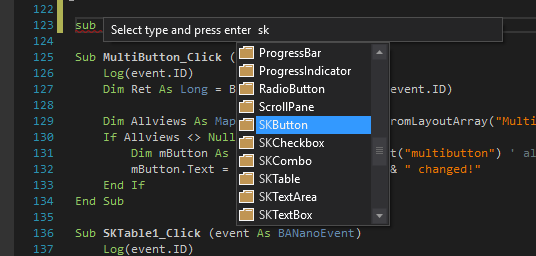

3. LOADING A LAYOUT MULTIPLE TIMES
Sometimes, you are going to want to re-use a certain layout multiple times. This can for example be because you made a layout for a list item, and now want to re-use it for each item in your list.
This can be done using the BANano.LoadLayoutArray method:
B4X:
' loading layouts as array (multiple times loading the same layout)
For i = 0 To 4
Dim Ret As Long
Dim AllViews As Map
Ret = BANano.LoadLayoutArray("#r3", "MultiLayout", (i=0)) ' only clear the parent if it is the first layout that is loaded
' ret returns a unique number you can use to get all views
AllViews = BANano.GetAllViewsFromLayoutArray("MultiLayout", Ret)
Dim mLabel As SKLabel = AllViews.Get("multilabel") ' always lowercase
mLabel.Text = "I'm {C:#FF0000}{U}row " & (i+1) & "{/U}{/C} of a multi layout!"
Dim mButton As SKButton = AllViews.Get("multibutton") ' always lowercase
mButton.Text = "Multi Button " & Ret
Next
Nothing difficult here. The final parameter in LoadLayoutArray() can be used to clear the parent on which you are loading the layout (in this case #r3).
The method does return a 'unique' number. This is very useful to get all the views from your layout. We do this with the BANano.GetAllViewsFromLayoutArray() method.
The GetAllViewsFromLayoutArray() method returns a map with all the Views in it, for that layout, with instance 'unique number'.
So you can just grab Views and start manipulating them.
NOTE: you may think you should 'buffer' this AllViews in a map yourself but this is not needed! In the generated Javascript, this will already be done for you so you would do it twice.
There is also a 'helper' method BANano.GetSuffixFromID() to know what this 'unique' number is, in case for example you want to make changes further in your code in a certain event.
B4X:
Sub MultiButton_Click (event As BANanoEvent)
Log(event.ID)
Dim Ret As Long = BANano.GetSuffixFromID(event.ID)
Dim Allviews As Map = BANano.GetAllViewsFromLayoutArray("MultiLayout", Ret)
If Allviews <> Null Then
Dim mButton As SKButton = Allviews.Get("multibutton") ' always lowercase
mButton.Text = "Multi Button " & Ret & " changed!"
End If
End Sub
NOTE:
Now with the support of the B4X Sender keyword, this is even easier:
B4X:
Sub MultiButton_Click (event As BANanoEvent)
Log(event.ID)
Dim Ret As Long = BANano.GetSuffixFromID(event.ID)
Dim mButton As SKButton = Sender
mButton.Text = "Multi Button " & Ret & " changed!"
End Sub
This concludes the 2 part tutorial of the new UI system in BAnano v2. Possibilities are endless and so much closer to standard B4J than the UI system v1. But, we learn of our mistakes
Alwaysbusy
Last edited: