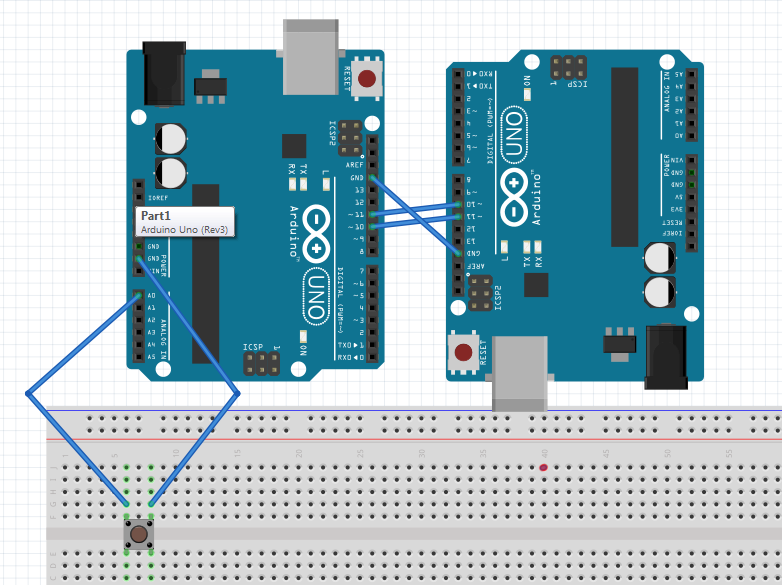
With the rSoftwareSerial library you can turn regular pins to serial ports. This is useful as the hardware serial is used by the IDE during development.
There are some limitations to the supported pins: https://www.arduino.cc/en/Reference/softwareSerial
As an example, the following program should run on two boards. When you press on the connected button, the on-board led on the other board will turn on.
B4X:
Sub Process_Globals
Public Serial1 As Serial
Private astream As AsyncStreams
Private led13 As Pin
Private btn As Pin
Private softserial As SoftwareSerial
End Sub
Private Sub AppStart
Serial1.Initialize(115200)
Log("appstart")
led13.Initialize(13, led13.MODE_OUTPUT)
led13.DigitalWrite(False)
softserial.Initialize(57600, 10, 11)
astream.Initialize(softserial.Stream, "astream_newdata", Null)
btn.Initialize(btn.A0, btn.MODE_INPUT_PULLUP)
btn.AddListener("Btn_StateChanged")
End Sub
Sub Btn_StateChanged (State As Boolean)
Dim b As Byte
If State Then b = 1 Else b = 0
astream.Write(Array As Byte(b))
End Sub
Sub astream_NewData (Buffer() As Byte)
Dim state As Boolean = Buffer(0) = 1 'state will be true if buffer(0) is 1.
led13.DigitalWrite(Not(state))
End Sub
The library is attached (it will be included in the next update).