Hello.
here is my attempt for a couple of classes to generate an (jQuery-)UI out of a WebApp.
With this classes you are able to open dialogs and place controls (buttons, textinputs, labels and more).
Here is a little demo: http://realsource.de:51234/
Example:
Please take a look into the Websocket-Files (those who begin with ws*) for further explanations
I'm sure, that the code can be optimized. Therefore i will be glad about constructive criticism.
Greetings ... Peter
Update 2014-05-20: Add Slider, Progressbar (thanks to flapjacks), BlockUI. Minor improvements.
Update 2014-06-20: Add Google Chart and some Client-Events (MouseMove and Resize). Minor improvements.
Update 2014-06-21: Fixed UICheckBox (Thanks to Christos Dorotheou)
here is my attempt for a couple of classes to generate an (jQuery-)UI out of a WebApp.
With this classes you are able to open dialogs and place controls (buttons, textinputs, labels and more).
Here is a little demo: http://realsource.de:51234/
Example:
B4X:
'WebSocket class
Sub Class_Globals
Private WS As WebSocket
Dim dlgHelloWorld As UIDialog
Dim cmdHelloWorld As UIButton
End Sub
Private Sub WebSocket_Connected(WebSocket1 As WebSocket)
WS = WebSocket1
dlgHelloWorld.Initialize(WS, "dlgHelloWorld", True) ' Create a modal dialog
dlgHelloWorld.Resize(-1, -1, 800, 500)
dlgHelloWorld.SetTitle("HelloWorld")
dlgHelloWorld.Open
cmdHelloWorld.Initialize(WS, "cmdHelloWorld")
cmdHelloWorld.SetText("HelloWorld")
cmdHelloWorld.appendTo("dlgHelloWorld")
End Sub
Private Sub cmdHelloWorld_Click(Params As Map)
WS.Alert("Hello World!")
End Sub
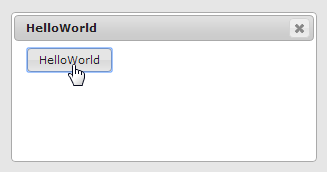
Please take a look into the Websocket-Files (those who begin with ws*) for further explanations
I'm sure, that the code can be optimized. Therefore i will be glad about constructive criticism.
Greetings ... Peter
Update 2014-05-20: Add Slider, Progressbar (thanks to flapjacks), BlockUI. Minor improvements.
Update 2014-06-20: Add Google Chart and some Client-Events (MouseMove and Resize). Minor improvements.
Update 2014-06-21: Fixed UICheckBox (Thanks to Christos Dorotheou)
Attachments
Last edited: