The ABMChronologyList is a vertical timeline component. Useful to give an overview of a limited period. It is device aware so e.g. on a phone, all items will be one under each other.
Usage
Create some themes:
Add the component and add some 'slides':
The helper method, BuildFiche():
Using events to change the 'status' of a slide:
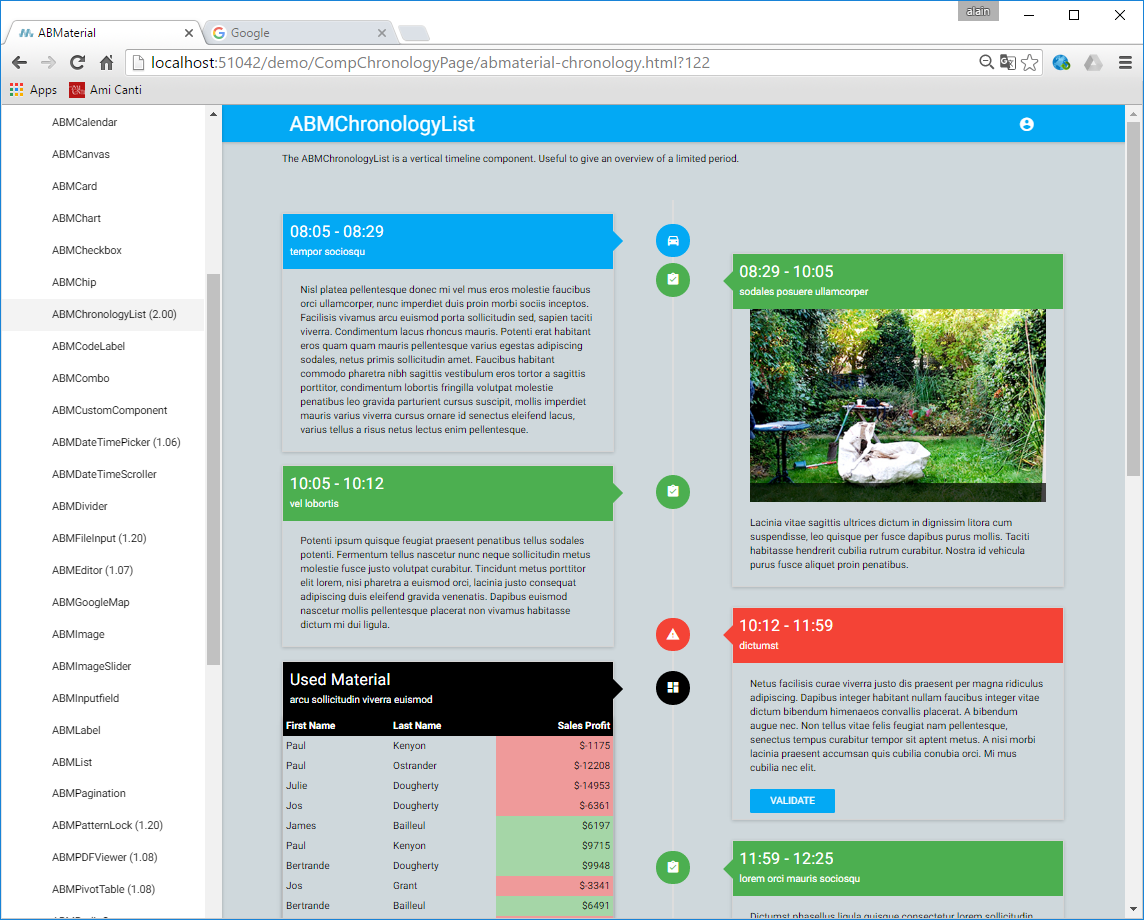
Usage
Create some themes:
B4X:
theme.AddChronologyList("chrono")
theme.ChronologyList("chrono").AddChronologyBadgeTheme("blue")
theme.ChronologyList("chrono").ChronologyBadge("blue").BackColor = ABM.COLOR_LIGHTBLUE
theme.ChronologyList("chrono").ChronologyBadge("blue").ColorizePointer = True
theme.ChronologyList("chrono").ChronologyBadge("blue").IconName = "mdi-maps-directions-car"
theme.ChronologyList("chrono").AddChronologyBadgeTheme("red")
theme.ChronologyList("chrono").ChronologyBadge("red").BackColor = ABM.COLOR_RED
theme.ChronologyList("chrono").ChronologyBadge("red").ColorizePointer = True
theme.ChronologyList("chrono").ChronologyBadge("red").IconName = "mdi-action-report-problem"
theme.ChronologyList("chrono").AddChronologyBadgeTheme("green")
theme.ChronologyList("chrono").ChronologyBadge("green").BackColor = ABM.COLOR_GREEN
theme.ChronologyList("chrono").ChronologyBadge("green").ColorizePointer = True
theme.ChronologyList("chrono").ChronologyBadge("green").IconName = "mdi-action-assignment-turned-in"
theme.ChronologyList("chrono").AddChronologyBadgeTheme("black")
theme.ChronologyList("chrono").ChronologyBadge("black").BackColor = ABM.COLOR_BLACK
theme.ChronologyList("chrono").ChronologyBadge("black").ColorizePointer = True
theme.ChronologyList("chrono").ChronologyBadge("black").IconName = "mdi-action-dashboard"
Add the component and add some 'slides':
B4X:
Dim chrono As ABMChronologyList
chrono.Initialize(page, "chrono", "chrono")
chrono.AddSlide("Slide1", BuildFiche(1, "08:05 - 08:29", "", "blue"), "blue")
chrono.AddSlide("Slide2", BuildFiche(2, "08:29 - 10:05", "../images/gardenbefore.jpg", "green"), "green")
chrono.AddSlide("Slide3", BuildFiche(3, "10:05 - 10:12", "", "green"), "green")
chrono.AddSlide("Slide4", BuildFiche(4, "10:12 - 11:59", "", "red"), "red")
chrono.AddSlide("Slide5", BuildFiche(5, "Used Material", "", "black"), "black")
chrono.AddSlide("Slide6", BuildFiche(6, "11:59 - 12:25", "", "green"), "green")
chrono.AddSlide("Slide7", BuildFiche(7, "12:25 - 15:02", "", "green"), "green")
chrono.AddSlide("Slide8", BuildFiche(8, "15:02 - 15:17", "", "green"), "green")
chrono.AddSlide("Slide9", BuildFiche(9, "15:17 - 16:32", "", "red"), "red")
chrono.AddSlide("Slide10", BuildFiche(10, "16:32 - 17:43", "../images/gardenafter.jpg", "green"), "green")
chrono.AddSlide("Slide11", BuildFiche(11, "17:43 - 18:07", "", "blue"), "blue")
page.Cell(2,1).AddComponent(chrono)
The helper method, BuildFiche():
B4X:
Sub BuildFiche(id As String, Title As String, pic As String, themeName As String) As ABMContainer
Dim Fiche As ABMContainer
Fiche.Initialize(page, "fiche" & id, "")
Fiche.AddRowsM(1, False,0,0, themeName).AddCellsOSMP(1,0,0,0,12,12,12,0,12,10,10,"")
If themeName = "black" Then
Fiche.AddRowsM(1, False,0,0, "").AddCellsOSMP(1,0,0,0,12,12,12,0,0,0,0,"")
Else
Fiche.AddRowsM(1, True,0,0, "").AddCellsOSMP(1,0,0,0,12,12,12,0,0,0,0,"")
End If
If themeName = "red" Then
Fiche.AddRowsM(1, True,0,10, "").AddCellsOSMP(1,0,0,0,12,12,12,0,0,0,0,"")
End If
Fiche.BuildGrid
If themeName = "black" Then
Fiche.Row(2).MarginLeft = "auto"
Fiche.Row(2).MarginRight = "auto"
End If
Fiche.Row(1).MarginLeft = "0"
Fiche.Row(1).MarginRight = "0"
Dim titLabel As ABMLabel
titLabel.Initialize(page, "titlabel" & id, Title, ABM.SIZE_H5,False, "white")
Fiche.Cell(1,1).AddComponent(titLabel)
Dim titLabel2 As ABMLabel
titLabel2.Initialize(page, "titlabel2_" & id, ABM.Util.words(Rnd(1,5)), ABM.SIZE_H6,False, "white")
Fiche.Cell(1,1).AddComponent(titLabel2)
If pic <> "" Then
Fiche.Cell(2,1).AddComponent(ABMShared.BuildImage(page, "ficheimg" & id, pic,1, "Garden"))
End If
If themeName = "black" Then
' create a table
Dim tbl1 As ABMTable
tbl1.Initialize(page, "tbl1", True, False, True, "tbl1theme")
tbl1.SetHeaders(Array As String("First Name", "Last Name", "Sales Profit"))
tbl1.SetHeaderThemes(Array As String("headerfooter", "headerfooter", "headerfooterright"))
Dim FirstNames As List
Dim LastNames As List
FirstNames.Initialize2(Array As String("Alain", "Jos", "Paul", "Bertrande", "Julie", "Stephanie", "Bert", "Charles", "James", "Walter"))
LastNames.Initialize2(Array As String("Bailleul", "Rogiers", "Dougherty", "Rodrigues", "Ostrander", "Kenyon", "Grant", "Klinger", "Sheffield", "Pointdexter"))
' add some random values
For i = 0 To 9
Dim r As List
Dim rCellThemes As List
r.Initialize
rCellThemes.Initialize
r.Add(FirstNames.get(Rnd(0,9)))
rCellThemes.Add("nocolor")
r.Add(LastNames.get(Rnd(0,9)))
rCellThemes.Add("nocolor")
Dim Neg As Int = Rnd(0,100)
If Neg > 50 Then
r.Add("$" & Rnd(1000, 15000))
rCellThemes.Add("positive")
Else
r.Add("$-" & Rnd(1000, 15000))
rCellThemes.Add("negative")
End If
tbl1.AddRow("uid" & i, r)
tbl1.SetRowThemes(rCellThemes) ' make sure you have as many items in rCellThemes as in r!
Next
tbl1.SetFooter("This is a footer that appears on the bottom of the table.", 12,"headerfooter")
Fiche.Cell(2,1).AddComponent(tbl1)
Else
Dim body As ABMLabel
body.Initialize(page, "body" & id, ABM.Util.sentences(Rnd(1,10)), ABM.SIZE_PARAGRAPH, True, "")
Fiche.Cell(2,1).AddComponent(body)
End If
If themeName = "red" Then
Dim btn As ABMButton
btn.InitializeFlat(page, id, "", "", "Validate", "")
Fiche.Cell(3,1).AddArrayComponent(btn, "btn")
End If
Return Fiche
End Sub
Using events to change the 'status' of a slide:
B4X:
Sub btn_Clicked(Target As String)
Dim slideNr As String = ABMShared.Mid2(Target,4)
Dim chrono As ABMChronologyList = page.Component("chrono")
chrono.SetSlideTheme("Slide" & slideNr, "green")
chrono.GetSlideContainer("Slide" & slideNr).Cell(3,1).RemoveAllComponents
chrono.GetSlideContainer("Slide" & slideNr).Row(1).UseTheme("green")
chrono.Refresh
End Sub
Last edited: