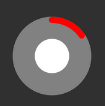
Using Kotlin libraries is similar to using Java libraries. The only difference is that we need to add references to two additional libraries:
http://central.maven.org/maven2/org/jetbrains/kotlin/kotlin-stdlib/1.3.21/kotlin-stdlib-1.3.21.jar
http://central.maven.org/maven2/org...lib-jdk7/1.3.21/kotlin-stdlib-jdk7-1.3.21.jar
B4X:
#AdditionalJar: kotlin-stdlib-1.3.21
#AdditionalJar: kotlin-stdlib-jdk7-1.3.21
As an example, I wanted to show how to use this open source project, without writing a wrapper: https://github.com/iammert/CameraVideoButton
The first step is to get the jar or AAR library from the maven repository. I like the direct approach. (The indirect approach is to create an Android Studio project based on the instructions and then find the downloaded resource.)
1. Get the compiled library
The setup instructions from that page:
B4X:
allprojects {
repositories {
...
maven { url 'https://jitpack.io' }
}
}
dependencies {
implementation 'com.github.iammert:CameraVideoButton:0.2'
}
https://jitpack.io/com/github/iammert/CameraVideoButton/0.2/
Follow this link to see the list of files available.
We can then download the aar file: https://jitpack.io/com/github/iammert/CameraVideoButton/0.2/CameraVideoButton-0.2.aar
The other interesting file is the POM file. It lists the other dependencies. Note that the other dependencies might depend on more resources.
2. Calling the constructor
We need to look in the code and find the constructor: https://github.com/iammert/CameraVi...ary/cameravideobuttonlib/CameraVideoButton.kt
The B4A code:
B4X:
Dim ctxt As JavaObject
ctxt.InitializeContext
CameraVideoButton.InitializeNewInstance("com.iammert.library.cameravideobuttonlib.CameraVideoButton", _
Array(ctxt, Null, 0))
Once we see that it is working we translate the example code and also create the listener:
B4X:
Dim duration As Long = 10000
CameraVideoButton.RunMethod("setVideoDuration", Array(duration))
CameraVideoButton.RunMethod("enableVideoRecording", Array(True))
CameraVideoButton.RunMethod("enablePhotoTaking", Array(True))
Dim listener As Object = CameraVideoButton.CreateEventFromUI("com.iammert.library.cameravideobuttonlib.CameraVideoButton.ActionListener", _
"VideoListener", Null)
CameraVideoButton.RunMethod("setActionListener", Array(listener))
Activity.AddView(CameraVideoButton, 50%x - 50dip, 100%y - 120dip, 100dip, 100dip)
Sub VideoListener_Event (MethodName As String, Args() As Object) As Object
Log(MethodName)
Return Null
End Sub
Note that the duration parameter type is Long. I first wrote Array(10000) and it failed with a class cast exception.
At this point everything worked but I wasn't able to change the "recording color" value. The library doesn't provide a method to set it programmatically. It is extracted from the view's XML declaration:
B4X:
recordingColor = typedArray.getColor(R.styleable.CameraVideoButton_cvb_recording_color, Color.WHITE)
We can set it by creating a custom theme with the manifest editor:
B4X:
SetApplicationAttribute(android:theme, "@style/Theme")
CreateResource(values, theme.xml,
<resources>
<style name="Theme" parent="@android:style/Theme.Material">
<item name="cvb_recording_color">#ffff0000</item>
</style>
</resources>
)
The project is attached.
Attachments
Last edited: