JSch is an open source Java implementation of SSH2. It supports many features.
Currently the Basic4android JSch library supports the SFTP protocol which is SSH File Transfer Protocol or Secured File Transfer Protocol.
SFTP is similar to FTP with the difference that the communication is done over a secured channel.
A good tutorial about SSH authentication is available here: SSH Host Key Protection | Symantec Connect Community
When the client first connects to the SSH server he needs to approve the host key (unless it is in the list of approved keys).
The SFtp object raises a PromptYesNo event for this question:
The network thread will wait until you call SetPromptResult or after 60 seconds. In the later case the result will be False.
If you want to automatically accept the host key (which should only be done in a secured local network) then you can write:
Sftp should be declared as a process global variable and initialized in Activity_Create (or Service_Create):
Calling SetKnownHostsStore sets a file that will save the known keys. Without it the user will need to approve the key each time.
In Activity_Resume we need to call SFtp.Activity_Resume:
The purpose of this call is to redisplay the visible prompt if it was visible when the activity was paused. This is relevant for example when the user changes the orientation while the dialog is visible.
SFtp can also show a message to the user in some cases. This is done with the ShowMessage event:
The other methods work in the same way as the FTP object: Android FTP tutorial
The library is attached. The open source project is embedded in the jar file.
Updates:
1.31 - New Rmdir method - deletes empty folders.
1.30 - Based on the latest version of Jsch (0.1.54).
Currently the Basic4android JSch library supports the SFTP protocol which is SSH File Transfer Protocol or Secured File Transfer Protocol.
SFTP is similar to FTP with the difference that the communication is done over a secured channel.
A good tutorial about SSH authentication is available here: SSH Host Key Protection | Symantec Connect Community
When the client first connects to the SSH server he needs to approve the host key (unless it is in the list of approved keys).
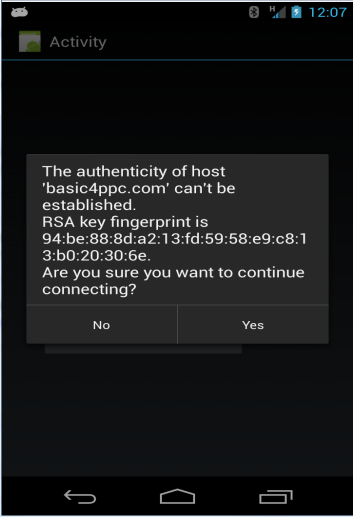
The SFtp object raises a PromptYesNo event for this question:
B4X:
Sub sftp1_PromptYesNo (Message As String)
Dim res As Int = Msgbox2(Message, "", "Yes", "", "No", Null)
'The next line might be a bit confusing. It is a condition.
'The value will be True if res equals to DialogResponse.POSITIVE.
sftp1.SetPromptResult(res = DialogResponse.POSITIVE)
End Sub
If you want to automatically accept the host key (which should only be done in a secured local network) then you can write:
B4X:
Sub sftp1_PromptYesNo (Message As String)
sftp1.SetPromptResult(True)
End Sub
Sftp should be declared as a process global variable and initialized in Activity_Create (or Service_Create):
B4X:
Sub Activity_Create(FirstTime As Boolean)
If FirstTime Then
sftp1.Initialize("sftp1", "username", "password", "example.com", 22)
sftp1.SetKnownHostsStore(File.DirInternal, "hosts.txt")
End If
Activity.LoadLayout("1")
End Sub
Calling SetKnownHostsStore sets a file that will save the known keys. Without it the user will need to approve the key each time.
In Activity_Resume we need to call SFtp.Activity_Resume:
B4X:
Sub Activity_Resume
sftp1.Activity_Resume
End Sub
SFtp can also show a message to the user in some cases. This is done with the ShowMessage event:
B4X:
Sub sftp1_ShowMessage (Message As String)
Msgbox(Message, "")
End Sub
The other methods work in the same way as the FTP object: Android FTP tutorial
The library is attached. The open source project is embedded in the jar file.
Updates:
1.31 - New Rmdir method - deletes empty folders.
1.30 - Based on the latest version of Jsch (0.1.54).
Attachments
Last edited: