Wish: Compile B4X constants to native Java "finals"
Today B4X constants are implemented by the IDE/precompiler only. If you try to reassign a value to a constant it's the IDE that raises the error.
When B4X compiles the app to Java the B4X constants are converted to regular Java variables and thus do not benefit from the Java compiler's ability to optimize constants with inlining.
ABMaterial applications greatly benefit from the use of constants to give meaning to the dozens of parameters used for building the grid, navigationbar, themes, menus, and so on... Each ABM page may have dozens of API calls executed each time the page is refreshed.
While using constants adds clarity to ABM pages, this adds overhead to load the B4X constant variables. If they were compiled to native Java constants their would be little to no performance penalty.
This routine uses four B4X constants.
These could be optimized with Java finals.
Today B4X constants are implemented by the IDE/precompiler only. If you try to reassign a value to a constant it's the IDE that raises the error.
When B4X compiles the app to Java the B4X constants are converted to regular Java variables and thus do not benefit from the Java compiler's ability to optimize constants with inlining.
ABMaterial applications greatly benefit from the use of constants to give meaning to the dozens of parameters used for building the grid, navigationbar, themes, menus, and so on... Each ABM page may have dozens of API calls executed each time the page is refreshed.
While using constants adds clarity to ABM pages, this adds overhead to load the B4X constant variables. If they were compiled to native Java constants their would be little to no performance penalty.
This routine uses four B4X constants.
B4X:
'*------------------------------------------------------------------- BuildPageGrid
'*
Sub BuildPageGrid()
Private Const ROW_MARGIN_TOP=4, ROW_MARGIN_BOTTOM=4 As Int
Private Const CENTER_IN_ROW=True As Boolean
Private Const DEFAULT_THEME="" As String
Private mt, mb, pl, pr As Int
' -----------------------------------------------------
' Notes
'
' mt = margin top (px)
' mb = margin bot (px)
' pl = pad left (px)
' pr = pad right (px)
' -----------------------------------------------------
' 1. Category
' 2. Products
' 3. Project Dimensions
' 4. Length
' 5. Width
' 6. Depth
' -----------------------------------------------------
page.AddRowsM( 6, CENTER_IN_ROW, ROW_MARGIN_TOP, ROW_MARGIN_BOTTOM, DEFAULT_THEME ).AddCellsOS(1, 0, 0, 0, 11, 6, 6, DEFAULT_THEME).AddCellsOS(1, 0, 0, 0, 1, 6, 6, DEFAULT_THEME)
' -----------------------------------------------------
' 7. Amount
' -----------------------------------------------------
mt=2 : mb=0 : pl=16 : pr=0
page.AddRowsM( 1, CENTER_IN_ROW, ROW_MARGIN_TOP, ROW_MARGIN_BOTTOM, DEFAULT_THEME ).AddCellsOSMP(1, 0, 0, 0, 11, 6, 6, mt, mb, pl, pr, DEFAULT_THEME).AddCellsOSMP(1, 0, 0, 0, 1, 6, 6, 0, 0, 0, 0,DEFAULT_THEME)
' -----------------------------------------------------
' 8. Calculate
' -----------------------------------------------------
mt=12 : mb=0 : pl=0 : pr=0
page.AddRowsM( 1, CENTER_IN_ROW, ROW_MARGIN_TOP, ROW_MARGIN_BOTTOM, DEFAULT_THEME ).AddCellsOSMP(1, 0, 0, 0, 11, 6, 6, mt, mb, pl, pr, DEFAULT_THEME).AddCellsOSMP(1, 0, 0, 0, 1, 6, 6, 0, 0, 0, 0,DEFAULT_THEME)
' -----------------------------------------------------
' 9. Blank row
' 10. Blank row
' -----------------------------------------------------
page.AddRowsM( 2, CENTER_IN_ROW, ROW_MARGIN_TOP, ROW_MARGIN_BOTTOM, DEFAULT_THEME ).AddCellsOS(1, 0, 0, 0, 11, 6, 6, DEFAULT_THEME).AddCellsOS(1, 0, 0, 0, 1, 6, 6, DEFAULT_THEME)
End Sub
These could be optimized with Java finals.
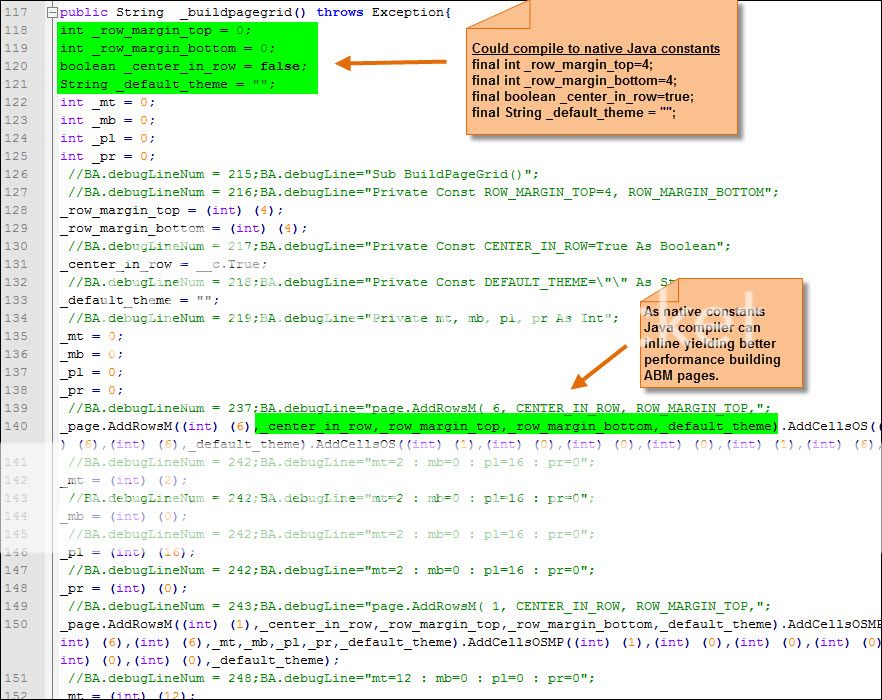
Last edited: