The online example is currently disabled due to spam 
Now that both B4A and B4J support WebSockets it is possible to implement a full push framework solution.
The solution is made of two components. The client app (B4A) and the server WebApp (B4J).
The client opens and maintains a WebSocket connection with the server.
In the browser you can see the number of active connections and the total number of users (which includes inactive users). You can send a message to all users.
The message is queued in a database and will be delivered to the devices when they connect.
A similar process happens on the device. If the activity is paused then the message is stored with the help of KeyValueStore and a notification is shown. Later when the activity becomes visible the messages are listed on the device.
Note that the device can also send messages to the server (it is not implemented in the demo interface).
It is also possible to send messages to specific ids.
Please give it a try. You need to first download WebSocket library (v1.01+): http://www.b4x.com/android/forum/threads/40221/#content
Run the program and then go to the online console to send a message: http://basic4ppc.com:51042/push/index.html
You can also download the compiled apk and install it.
The server code is available here: http://www.b4x.com/android/forum/threads/webapp-web-apps-overview.39811
B4J client implementation: http://www.b4x.com/android/forum/threads/jwebsocketclient-library.40985/
Edit: Both the server code and device code were updated.
This example can be the base for many types of solutions that require a persistent server connection.
Now that both B4A and B4J support WebSockets it is possible to implement a full push framework solution.
The solution is made of two components. The client app (B4A) and the server WebApp (B4J).
The client opens and maintains a WebSocket connection with the server.
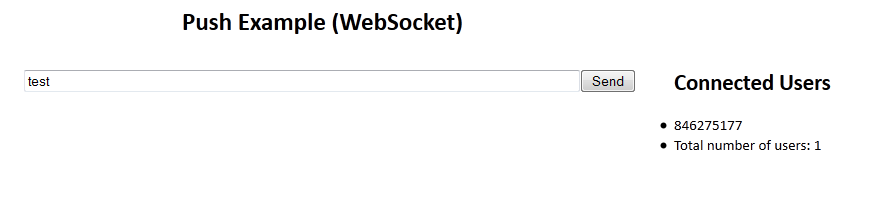
In the browser you can see the number of active connections and the total number of users (which includes inactive users). You can send a message to all users.
The message is queued in a database and will be delivered to the devices when they connect.
A similar process happens on the device. If the activity is paused then the message is stored with the help of KeyValueStore and a notification is shown. Later when the activity becomes visible the messages are listed on the device.
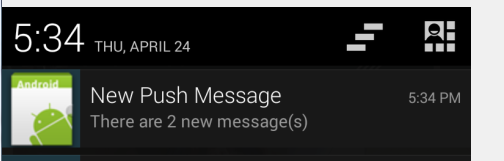

Note that the device can also send messages to the server (it is not implemented in the demo interface).
It is also possible to send messages to specific ids.
Please give it a try. You need to first download WebSocket library (v1.01+): http://www.b4x.com/android/forum/threads/40221/#content
Run the program and then go to the online console to send a message: http://basic4ppc.com:51042/push/index.html
You can also download the compiled apk and install it.
The server code is available here: http://www.b4x.com/android/forum/threads/webapp-web-apps-overview.39811
B4J client implementation: http://www.b4x.com/android/forum/threads/jwebsocketclient-library.40985/
Edit: Both the server code and device code were updated.
This example can be the base for many types of solutions that require a persistent server connection.
Attachments
Last edited: