Dialogs v4 is an extension to @agraham original dialogs library. It requires B4A v7+.
The main changes are:
- New CustomLayoutDialog which makes it easier to develop custom dialogs.
- New ShowAsync methods for all dialogs except of CustomDialog and CustomDialog2.
The ShowAsync methods behave similar to Msgbox2Async and the other internal async dialogs: DoEvents deprecated and async dialogs (msgbox)
Example:
The async methods also include a Cancelable parameter that determines whether the dialog can be cancelled with the back key or a click outside of the dialog.
There are also other small improvements in the async methods implementation.
As explained in the tutorial about DoEvents, it is recommended to switch to the async methods.
Do note that ShowAsync + Wait For behavior is not identical to the modal dialogs behavior. When Wait For is called, the code returns to the calling sub (if there is one). If you want that the calling sub will wait for the sub that shows the async dialog: https://www.b4x.com/android/forum/threads/b4x-resumable-subs-sleep-wait-for.78601/page-2#post-499130
CustomLayoutDialog
CustomLayoutDialog replaces CustomDialog and CustomDialog2. As it is a non-modal dialog, it is more powerful. You can handle events while the dialog is visible and manage the dialog itself.
Note that CustomLayoutDialog is supported by Android 4+ (API 14+).
Showing the dialog is done in two steps:
1. Call ShowAsync and wait for Dialog_Ready event.
2. Load the layout to the panel (or create it programmatically).
The Dialog_Result event will be raised when the dialog is closed.
You can close the dialog programmatically by calling CloseDialog with the result:
You can explicitly set the dialog's size by calling SetSize. You need to call it after calling ShowAsync and before the Dialog_Ready event.
Note that the size set is the size of the dialog. The panel itself will be smaller. Use a layout with anchors to make sure that the layout adapts properly.
Dialog.GetButton will return one of the dialogs buttons. It can be used to disable or enable a button.
For example this code enables the OK button when all fields are non-empty.
The example is attached.
The library is available here: https://www.b4x.com/android/forum/threads/dialogs-library.6776
Edit: Cross platform custom layout dialog based on XUI: https://www.b4x.com/android/forum/threads/99756/#content
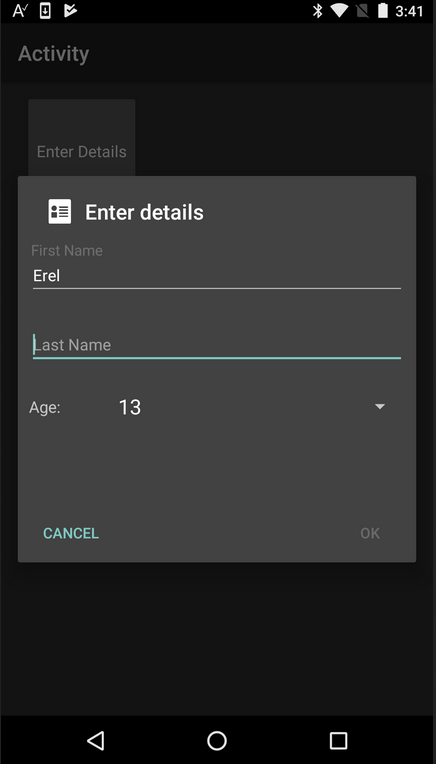
The main changes are:
- New CustomLayoutDialog which makes it easier to develop custom dialogs.
- New ShowAsync methods for all dialogs except of CustomDialog and CustomDialog2.
The ShowAsync methods behave similar to Msgbox2Async and the other internal async dialogs: DoEvents deprecated and async dialogs (msgbox)
Example:
B4X:
Dim cd As ColorDialog
Dim sf As Object = cd.ShowAsync("Choose Color", "Yes", "Cancel", "No", Null, False)
Wait For (sf) Dialog_Result(Result As Int)
If Result = DialogResponse.POSITIVE Then
Activity.Color = cd.RGB
End If
There are also other small improvements in the async methods implementation.
As explained in the tutorial about DoEvents, it is recommended to switch to the async methods.
Do note that ShowAsync + Wait For behavior is not identical to the modal dialogs behavior. When Wait For is called, the code returns to the calling sub (if there is one). If you want that the calling sub will wait for the sub that shows the async dialog: https://www.b4x.com/android/forum/threads/b4x-resumable-subs-sleep-wait-for.78601/page-2#post-499130
CustomLayoutDialog
CustomLayoutDialog replaces CustomDialog and CustomDialog2. As it is a non-modal dialog, it is more powerful. You can handle events while the dialog is visible and manage the dialog itself.
Note that CustomLayoutDialog is supported by Android 4+ (API 14+).
Showing the dialog is done in two steps:
1. Call ShowAsync and wait for Dialog_Ready event.
2. Load the layout to the panel (or create it programmatically).
The Dialog_Result event will be raised when the dialog is closed.
You can close the dialog programmatically by calling CloseDialog with the result:
B4X:
Dialog.CloseDialog (DialogResponse.POSITIVE)
You can explicitly set the dialog's size by calling SetSize. You need to call it after calling ShowAsync and before the Dialog_Ready event.
Note that the size set is the size of the dialog. The panel itself will be smaller. Use a layout with anchors to make sure that the layout adapts properly.
Dialog.GetButton will return one of the dialogs buttons. It can be used to disable or enable a button.
For example this code enables the OK button when all fields are non-empty.
B4X:
Sub DialogAge_ItemClick (Position As Int, Value As Object)
CheckAllFieldsValid
End Sub
Sub DialogLastName_TextChanged (Old As String, New As String)
CheckAllFieldsValid
End Sub
Sub DialogFirstName_TextChanged (Old As String, New As String)
CheckAllFieldsValid
End Sub
Sub CheckAllFieldsValid
Dim valid As Boolean = DialogAge.SelectedIndex > 0 And DialogFirstName.Text.Length > 0 And DialogLastName.Text.Length > 0
DetailsDialog.GetButton(DialogResponse.POSITIVE).Enabled = valid
End Sub
The example is attached.
The library is available here: https://www.b4x.com/android/forum/threads/dialogs-library.6776
Edit: Cross platform custom layout dialog based on XUI: https://www.b4x.com/android/forum/threads/99756/#content
Attachments
Last edited: