It is simpler to parse XML with Xml2Map class: https://www.b4x.com/android/forum/threads/b4x-xml2map-simple-way-to-parse-xml-documents.74848/
The XmlSax library provides an XML Sax parser.
This parser sequentially reads the stream and raises events at the beginning and end of each element.
The developer is responsible to do something useful with those events.
There are two events:
The StartElement is raised when an element begins. This event includes the element's attributes list.
EndElement is raised when an element ends. This event includes the element's text.
In this example we will parse the forum RSS feed. RSS is formatted using XML.
A simplified example of this RSS is:
The first line is part of the XML protocol and is ignored.
On the second line the StartElement event will be raised with "Name = rss" and the attributes will include the "version" field.
The EndElement of the rss element will only be called on the last line: </rss>.
We will populate a list view with all items parsed from an offline file. When the user will press on an item we will open the browser with the relevant link.
Every item represents a forum thread.
For each item we are interested in two values. The title and the link.
The SaxParser object includes a handy list that holds the names of all the current parents elements.
This is useful as it will help us find the "correct" 'title' and 'link' elements. The correct elements are the ones under the 'item' element.
The parsing code in this case is pretty simple:
Title and Link are global variables.
We are only using EndElement events in this program.
First we check if we are inside an 'item' element. If this is the case we check the actual element name and save it if it is 'title' or 'link'.
If the current element is 'item' it means that we are done parsing an item.
So we add the data collected to the list view.
We are using ListView.AddSingleLine2. This method receives two values. The first is the item text and the second is the value that will return when the user will click on this item. In this case we are storing the link as the return value.
Later we will use it to open the browser:
The code that initiated the parsing is:
The XmlSax library provides an XML Sax parser.
This parser sequentially reads the stream and raises events at the beginning and end of each element.
The developer is responsible to do something useful with those events.
There are two events:
B4X:
StartElement (Uri As String, Name As String, Attributes As Attributes)
EndElement (Uri As String, Name As String, Text As StringBuilder)
EndElement is raised when an element ends. This event includes the element's text.
In this example we will parse the forum RSS feed. RSS is formatted using XML.
A simplified example of this RSS is:
B4X:
<?xml version="1.0" encoding="ISO-8859-1"?>
<rss version="2.0">
<channel>
<title>Basic4ppc / Basic4android - Android programming</title>
<link>http://www.b4x.com/forum</link>
<description>Basic4android - android programming and development</description>
<ttl>60</ttl>
<image>
<url>http://www.b4x.com/forum/images/misc/rss.jpg</url>
<title>Basic4ppc / Basic4android - Android programming</title>
<link>http://www.b4x.com/forum</link>
</image>
<item>
<title>Phone library was updated - V1.10</title>
<link>http://www.b4x.com/forum/additional-libraries-official-updates/6859-phone-library-updated-v1-10-a.html</link>
<pubDate>Sun, 12 Dec 2010 09:27:38 GMT</pubDate>
<guid isPermaLink="true">http://www.b4x.com/forum/additional-libraries-official-updates/6859-phone-library-updated-v1-10-a.html</guid>
</item>
...MORE ITEMS HERE
</channel>
</rss>
On the second line the StartElement event will be raised with "Name = rss" and the attributes will include the "version" field.
The EndElement of the rss element will only be called on the last line: </rss>.
We will populate a list view with all items parsed from an offline file. When the user will press on an item we will open the browser with the relevant link.
Every item represents a forum thread.
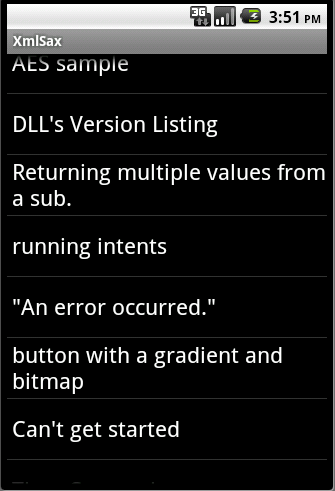
For each item we are interested in two values. The title and the link.
The SaxParser object includes a handy list that holds the names of all the current parents elements.
This is useful as it will help us find the "correct" 'title' and 'link' elements. The correct elements are the ones under the 'item' element.
The parsing code in this case is pretty simple:
B4X:
Sub Parser_StartElement (Uri As String, Name As String, Attributes As Attributes)
End Sub
Sub Parser_EndElement (Uri As String, Name As String, Text As StringBuilder)
If parser.Parents.IndexOf("item") > -1 Then
If Name = "title" Then
Title = Text.ToString
Else If Name = "link" Then
Link = Text.ToString
End If
End If
If Name = "item" Then
ListView1.AddSingleLine2(Title, Link) 'add the title as the text and the link as the value
End If
End Sub
We are only using EndElement events in this program.
First we check if we are inside an 'item' element. If this is the case we check the actual element name and save it if it is 'title' or 'link'.
If the current element is 'item' it means that we are done parsing an item.
So we add the data collected to the list view.
We are using ListView.AddSingleLine2. This method receives two values. The first is the item text and the second is the value that will return when the user will click on this item. In this case we are storing the link as the return value.
Later we will use it to open the browser:
B4X:
Sub ListView1_ItemClick (Position As Int, Value As Object)
StartActivity(PhoneIntents1.OpenBrowser(Value)) 'open the brower with the link
End Sub
B4X:
Dim in As InputStream
in = File.OpenInput(File.DirAssets, "rss.xml") 'This file was added with the file manager.
parser.Parse(in, "Parser") '"Parser" is the events subs prefix.
in.Close
Attachments
Last edited: