This is an old tutorial. All new implementations of network solutions should be based on AsyncStreams.
The Network library allows you to communicate over TCP/IP with other computers or devices.
The Network library contains two objects. Socket and ServerSocket.
The Socket object is the communication endpoint. Reading and writing are done with Socket.InputStream and Socket.OutputStream.
ServerSocket is an object that listens for incoming connections. Once a connection is established an event is raised and a socket object is passed to the event sub. This socket will be used to handle the new client.
Client application
Steps required:
- Create and initialize a Socket object.
- Call Socket.Connect with the server address.
- Connection is done in the background. The Connected event is raised when the connection is ready or if it failed.
- Communicate with the other machine using Socket.InputStream to read data and Socket.OutputStream to write data.
Server application
Steps required:
- Create and initialize a ServerSocket object.
- Call ServerSocket.Listen to listen for incoming connections. This happens in the background.
- Once a connection is established the NewConnection event is raised and a Socket object is passes.
- Call ServerSocket.Listen if you want to accept more connections.
- Using the Socket object received, communicate with the client.
We will see two examples.
The first example connects to a time server and displays the current date and time as received from the server.
We are creating a new socket and trying to connect to the server which is listening on port 13.
The next step is to wait for the Connected event.
If the connection is successful we create a TextReader object and initialize it with Socket1.InputStream. In this case we want to read characters and not bytes so a TextReader is used.
Calling tr.ReadLine may block. However we want to read at least a single line so it is fine.
Then we read all the other available lines (tr.Ready means that there is data in the buffer).
In the second application we will create a file transfer application, that will copy files from the desktop to the device.
The device will use a ServerSocket to listen to incoming connections.
Once a connection has been made, we will enable a timer. This timer checks every 200ms whether there is any data waiting to be read.
The file is sent in a specific protocol. First the file name is sent and then the actual file.
We are using a RandomAccessFile object to convert the bytes read to numeric values. RandomAccessFile can work with files or arrays of bytes, we are using the later in this case.
RandomAccessFile can be set to use little endian byte order. This is important here as the desktop uses this byte order as well.
The desktop example application was written with Basic4ppc.
Once connected the user selects a file and the file is sent to the device which saves it under /sdcard/android.
Both applications are attached.
Some notes about the code:
- The server is set to listen on port 2222.
The server displays its IP when it starts. The desktop client should use this IP address when connecting to a real device (this IP will not work with the emulator).
However if you work with the emulator or if your device is connected to the computer in debug mode you can use 'adb' to forward a desktop localhost port to the device.
This is done by issuing "adb forward tcp:5007 tcp:2222"
Now in the client code we should connect to the localhost ip with port 5007.
Again if you are testing this application in the emulator you must first run this adb command. Adb is part of the Android SDK.
- Listening to connections:
In Sub Activity_Resume (which also called right after Activity_Create) we call ServerSocket.Listen and start listening to connections. Note that you can call this method multiple times safely.
In Sub Activity_Pause we close the active connection (if there is such a connection) and also stop listening. This only happens if the user pressed on the back key (UserClosed = True).
The ServerSocket will later be initialized in Activity_Create.
The server side application can handle new connections. It will just replace the previous connection with the new one.
The desktop client example application doesn't handle broken connections. You will need to restart it to reconnect.
Edit: It is recommended to use the new AsnycStreams object instead of polling the available bytes parameter with a timer. Using AsyncStreams is simpler and more reliable.
The Network library allows you to communicate over TCP/IP with other computers or devices.
The Network library contains two objects. Socket and ServerSocket.
The Socket object is the communication endpoint. Reading and writing are done with Socket.InputStream and Socket.OutputStream.
ServerSocket is an object that listens for incoming connections. Once a connection is established an event is raised and a socket object is passed to the event sub. This socket will be used to handle the new client.
Client application
Steps required:
- Create and initialize a Socket object.
- Call Socket.Connect with the server address.
- Connection is done in the background. The Connected event is raised when the connection is ready or if it failed.
- Communicate with the other machine using Socket.InputStream to read data and Socket.OutputStream to write data.
Server application
Steps required:
- Create and initialize a ServerSocket object.
- Call ServerSocket.Listen to listen for incoming connections. This happens in the background.
- Once a connection is established the NewConnection event is raised and a Socket object is passes.
- Call ServerSocket.Listen if you want to accept more connections.
- Using the Socket object received, communicate with the client.
We will see two examples.
The first example connects to a time server and displays the current date and time as received from the server.
B4X:
Sub Process_Globals
Dim Socket1 As Socket
End Sub
Sub Globals
End Sub
Sub Activity_Create(FirstTime As Boolean)
Socket1.Initialize("Socket1")
Socket1.Connect("nist1-ny.ustiming.org" , 13, 20000)
End Sub
Sub Socket1_Connected (Successful As Boolean)
If Successful = False Then
Msgbox(LastException.Message, "Error connecting")
Return
End If
Dim tr As TextReader
tr.Initialize(Socket1.InputStream)
Dim sb As StringBuilder
sb.Initialize
sb.Append(tr.ReadLine) 'read at least one line
Do While tr.Ready
sb.Append(CRLF).Append(tr.ReadLine)
Loop
Msgbox("Time received: " & CRLF & sb.ToString, "")
Socket1.Close
End Sub
The next step is to wait for the Connected event.
If the connection is successful we create a TextReader object and initialize it with Socket1.InputStream. In this case we want to read characters and not bytes so a TextReader is used.
Calling tr.ReadLine may block. However we want to read at least a single line so it is fine.
Then we read all the other available lines (tr.Ready means that there is data in the buffer).
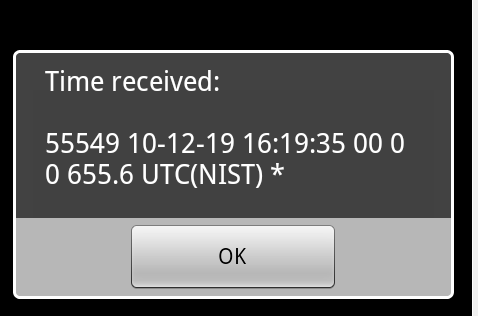
In the second application we will create a file transfer application, that will copy files from the desktop to the device.
The device will use a ServerSocket to listen to incoming connections.
Once a connection has been made, we will enable a timer. This timer checks every 200ms whether there is any data waiting to be read.
The file is sent in a specific protocol. First the file name is sent and then the actual file.
We are using a RandomAccessFile object to convert the bytes read to numeric values. RandomAccessFile can work with files or arrays of bytes, we are using the later in this case.
RandomAccessFile can be set to use little endian byte order. This is important here as the desktop uses this byte order as well.
The desktop example application was written with Basic4ppc.
Once connected the user selects a file and the file is sent to the device which saves it under /sdcard/android.
Both applications are attached.
Some notes about the code:
- The server is set to listen on port 2222.
The server displays its IP when it starts. The desktop client should use this IP address when connecting to a real device (this IP will not work with the emulator).
However if you work with the emulator or if your device is connected to the computer in debug mode you can use 'adb' to forward a desktop localhost port to the device.
This is done by issuing "adb forward tcp:5007 tcp:2222"
Now in the client code we should connect to the localhost ip with port 5007.
B4X:
Client.Connect("127.0.0.1", 5007)
- Listening to connections:
B4X:
Sub Activity_Resume
ServerSocket1.Listen
End Sub
Sub Activity_Pause (UserClosed As Boolean)
If UserClosed Then
Timer1.Enabled = False
Socket1.Close
ServerSocket1.Close 'stop listening
End If
End Sub
Sub ServerSocket1_NewConnection (Successful As Boolean, NewSocket As Socket)
If Successful Then
Socket1 = NewSocket
Timer1.Enabled = True
InputStream1 = Socket1.InputStream
OutputStream1 = Socket1.OutputStream
ToastMessageShow("Connected", True)
Else
Msgbox(LastException.Message, "Error connecting")
End If
ServerSocket1.Listen 'Continue listening to new incoming connections
End Sub
In Sub Activity_Pause we close the active connection (if there is such a connection) and also stop listening. This only happens if the user pressed on the back key (UserClosed = True).
The ServerSocket will later be initialized in Activity_Create.
The server side application can handle new connections. It will just replace the previous connection with the new one.
The desktop client example application doesn't handle broken connections. You will need to restart it to reconnect.
Edit: It is recommended to use the new AsnycStreams object instead of polling the available bytes parameter with a timer. Using AsyncStreams is simpler and more reliable.
Attachments
Last edited: