The Animation library allows you to animate views.
These small animations are really nice and can affect the user overall impression of your application.
The attached program demonstrates the available types of animations which are:
Alpha - Causes a fading in or fading out effect.
Scale - The view's size smoothly changes.
Rotate - The view rotates.
Translate - The view moves to a different position.
Creating an animation is done in four steps:
- Declare the animation object.
- Initialize the object based on the required animation.
- Set the animation parameters (duration, repeat and repeat mode).
- Start the animation by calling Start with the target view.
In this program an animation is attached to each button as its Tag value.
All the buttons click events are caught with Sub Button_Click.
In this sub we take the attached animation from the sender button and start it.
The code for the first five animations and buttons:
We are using a temporary array to avoid writing duplicate code.
Setting RepeatCount to 1 means that each animation will play twice.
The REPEAT_REVERSE means that the second time the animation will play it will play backwards.
The animation attached to Button6 is made of 4 chained animations. The button moves down, then left, then up and then right back to the start.
We are using these animations:
In this case we do not want to repeat each animation.
Starting the animations:
Last part if the usage of AnimationEnd event to start the next animation for Button6:
This program looks really nice on a real device. It also works on the emulator but the animations are less smooth.
These small animations are really nice and can affect the user overall impression of your application.
The attached program demonstrates the available types of animations which are:
Alpha - Causes a fading in or fading out effect.
Scale - The view's size smoothly changes.
Rotate - The view rotates.
Translate - The view moves to a different position.
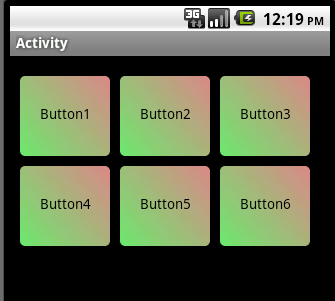
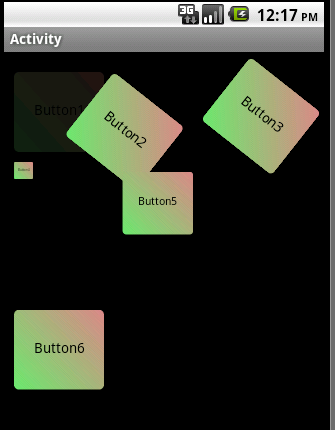
Creating an animation is done in four steps:
- Declare the animation object.
- Initialize the object based on the required animation.
- Set the animation parameters (duration, repeat and repeat mode).
- Start the animation by calling Start with the target view.
In this program an animation is attached to each button as its Tag value.
All the buttons click events are caught with Sub Button_Click.
In this sub we take the attached animation from the sender button and start it.
The code for the first five animations and buttons:
B4X:
Dim a1, a2, a3, a4, a5 As Animation
Activity.LoadLayout("1")
a1.InitializeAlpha("", 1, 0)
Button1.Tag = a1
a2.InitializeRotate("", 0, 180)
Button2.Tag = a2
a3.InitializeRotateCenter("", 0, 180, Button3)
Button3.Tag = a3
a4.InitializeScale("", 1, 1, 0, 0)
Button4.Tag = a4
a5.InitializeScaleCenter("", 1, 1, 0, 0, Button4)
Button5.Tag = a5
Dim animations() As Animation
animations = Array As Animation(a1, a2, a3, a4, a5)
For i = 0 To animations.Length - 1
animations(i).Duration = 1000
animations(i).RepeatCount = 1
animations(i).RepeatMode = animations(i).REPEAT_REVERSE
Next
Setting RepeatCount to 1 means that each animation will play twice.
The REPEAT_REVERSE means that the second time the animation will play it will play backwards.
The animation attached to Button6 is made of 4 chained animations. The button moves down, then left, then up and then right back to the start.
We are using these animations:
B4X:
a6.InitializeTranslate("Animation", 0, 0, 0dip, 200dip) 'we want to catch the AnimationEnd event for these animations
a7.InitializeTranslate("Animation", 0dip, 200dip, -200dip, 200dip)
a8.InitializeTranslate("Animation", -200dip, 200dip, -200dip, 0dip)
a9.InitializeTranslate("Animation", -200dip, 0dip, 0dip, 0dip)
Button6.Tag = a6
animations = Array As Animation(a6, a7, a8, a9)
For i = 0 To animations.Length - 1
animations(i).Duration = 500
Next
Starting the animations:
B4X:
Sub Button_Click
Dim b As Button
b = Sender
'Safety check. Not really required in this case.
If Not(b.Tag Is Animation) Then Return
Dim a As Animation
a = b.Tag
a.Start(b)
End Sub
B4X:
Sub Animation_AnimationEnd
If Sender = a6 Then
a7.Start(Button6)
Else If Sender = a7 Then
a8.Start(Button6)
Else If Sender = a8 Then
a9.Start(Button6)
End If
End Sub