A much improved version is available here.
You can use the code in this example to show data in tabular form.
The table is made of two main views. The header row is made of a panel with labels. The main cells component is made of a ScrollView with labels as the cells.
You can modify the code to change the table appearance.
Some of the settings can be changed in Sub Globals:
Adding data to the table:
Table events:
The code is not too complicated and you can further customize it as needed.
You can use the code in this example to show data in tabular form.
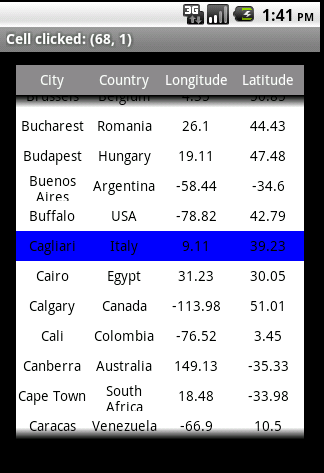
The table is made of two main views. The header row is made of a panel with labels. The main cells component is made of a ScrollView with labels as the cells.
You can modify the code to change the table appearance.
Some of the settings can be changed in Sub Globals:
B4X:
'Table settings
HeaderColor = Colors.Gray
NumberOfColumns = 4
RowHeight = 30dip
TableColor = Colors.White
FontColor = Colors.Black
HeaderFontColor = Colors.White
FontSize = 14
B4X:
'add header
SetHeader(Array As String("Col1", "Col2", "Col3", "Col4"))
'add rows
For i = 1 To 100
AddRow(Array As String(i, "Some text", i * 2, "abc"))
Next
'set the value of a specific cell
SetCell(0, 3, "New value")
'get the value
Log("Cell (1, 2) value = " & GetCell(1, 2))
B4X:
Sub Cell_Click
Dim rc As RowCol
Dim l As Label
l = Sender
rc = l.Tag
activity.Title = "Cell clicked: (" & rc.Row & ", " & rc.Col & ")"
End Sub
Sub Header_Click
Dim l As Label
Dim col As Int
l = Sender
col = l.Tag
Activity.Title = "Header clicked: " & col
End Sub