This is a Wrap for the Firebase UI-bindings found at Github.
It offers Authentification using
- Google-Account (Tested)
- email and password authentification (Tested)
- Telephone-Authentification (Tested)
- Twitter (Not tested as i do not have a Twitter-Dev Account)
- Facebook (Not tested as i do not have a Facebookr-Dev Account)
- Authentification using a Custom Token generated by the FirebaseAdmin SDK (B4J Library currently in Beta)
For the last two (Twitter and Facebook) there must be made additional changes to the Manifest.
Requirements:
- B4A 7.8+
- Manifestchanges needed!
- You app needs to be AppCompat enabled (must use AppCompat)
Mandatory:
- Prepare your app to use Firebase. https://www.b4x.com/android/forum/threads/integrating-firebase-services.67692/#content
- Google Play Services Base - Snippet
- Firebase Base - Snippet
- Firebase Auth - Snippet
- Additional Manifestentries for the firebase-UI bindings
Add this line to your Manifest to define the needed Firebase UI Activities.
- Mandatory additional Jars needed to the B4A Code
Basically you setup the allowed Auth methods with this Code:
NOTES:
- To use Google Authentification with this lib you need to provide the default_web_client id.
You can find the Value in the google-services.json
1. Open the google-services.json with Notepad++
2. Search for your apps packagename, you´ll find a snippet like this
4. Copy the value and use it in the getIdpGOOGLE_PROVIDER call above.
It will result in a Auth-Selection
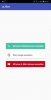
Using email
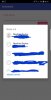
Using new email:
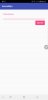
FirebaseUI
Author: DonManfred (wrapper)
Version: 0.6
The Library is free to use. You can however donate for it if you want
Note to download the additional JARs and AARs from here:
It offers Authentification using
- Google-Account (Tested)
- email and password authentification (Tested)
- Telephone-Authentification (Tested)
- Twitter (Not tested as i do not have a Twitter-Dev Account)
- Facebook (Not tested as i do not have a Facebookr-Dev Account)
- Authentification using a Custom Token generated by the FirebaseAdmin SDK (B4J Library currently in Beta)
For the last two (Twitter and Facebook) there must be made additional changes to the Manifest.
Requirements:
- B4A 7.8+
- Manifestchanges needed!
- You app needs to be AppCompat enabled (must use AppCompat)
Mandatory:
- Prepare your app to use Firebase. https://www.b4x.com/android/forum/threads/integrating-firebase-services.67692/#content
- Google Play Services Base - Snippet
- Firebase Base - Snippet
- Firebase Auth - Snippet
- Additional Manifestentries for the firebase-UI bindings
Add this line to your Manifest to define the needed Firebase UI Activities.
B4X:
CreateResourceFromFile(Macro, FirebaseAnalytics.GooglePlayBase)
CreateResourceFromFile(Macro, FirebaseAnalytics.Firebase)
CreateResourceFromFile(Macro, FirebaseUI.uibindings)
- Mandatory additional Jars needed to the B4A Code
B4X:
#AdditionalJar: androidx.legacy:legacy-support-core-utils
#AdditionalJar: androidx.annotation:annotation
#AdditionalJar: androidx.core:core
#AdditionalJar: androidx.appcompat:appcompat
#AdditionalJar: androidx.legacy:legacy-support-v4
#AdditionalJar: android.arch.lifecycle:extensions
#AdditionalJar: androidx.browser:browser
#AdditionalJar: androidx.cardview:cardview
#AdditionalJar: androidx.constraintlayout:constraintlayout
#AdditionalJar: com.google.android.material:material
#AdditionalJar: com.google.android.gms:play-services-auth
#AdditionalJar: com.google.firebase:firebase-core
#AdditionalJar: com.google.firebase:firebase-auth
#AdditionalJar: firebase-ui-auth-6.2.0.aar
#AdditionalJar: materialprogressbar-1.6.1.aar
#AdditionalRes: ..\res.FirebaseUI, com.firebase.ui.auth
Basically you setup the allowed Auth methods with this Code:
B4X:
Dim ib As SignInIntentBuilder
ib.Initialize("",fbauth.createSignInIntentBuilder)
ib.addProvider(ib.IdpEMAIL_PROVIDER)
ib.addProvider(ib.IdpPHONE_VERIFICATION_PROVIDER)
ib.addProvider(ib.IdpANONYMOUS_PROVIDER)
ib.addProvider(ib.getIdpGOOGLE_PROVIDER("xxxx-yyyy.apps.googleusercontent.com"))
'ib.addProvider(ib.IdpTWITTER_PROVIDER)
'ib.addProvider(ib.IdpFACEBOOK_PROVIDER)
Dim intent As Intent
intent = ib.setIsSmartLockEnabled2(False,True).setAvailableProviders(ib.Providerlist).setTosUrl("http://URLtoTOS").setPrivacyPolicyUrl("http://URLtoTOS").build
StartActivity(intent)
NOTES:
- To use Google Authentification with this lib you need to provide the default_web_client id.
You can find the Value in the google-services.json
1. Open the google-services.json with Notepad++
2. Search for your apps packagename, you´ll find a snippet like this
3. We are interested in the value of client_id from which client_type is 3."client_info": {
"mobilesdk_app_id": "1:888939569991:android:83f82a2b632ab8bf",
"android_client_info": {
"package_name": "de.donmanfred.fbui"
}
},
"oauth_client": [
{
"client_id": "dddd-ffff.apps.googleusercontent.com",
"client_type": 1,
"android_info": {
"package_name": "de.donmanfred.fbui",
"certificate_hash": "hhhh"
}
},
{
"client_id": "xxxx-yyyy.apps.googleusercontent.com",
"client_type": 3
}
4. Copy the value and use it in the getIdpGOOGLE_PROVIDER call above.
It will result in a Auth-Selection
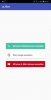
Using email
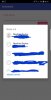
Using new email:
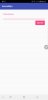
FirebaseUI
Author: DonManfred (wrapper)
Version: 0.6
- FirebaseOptions
- Functions:
- Initialize (apiKey As String, appID As String, dbUrl As String, GcmsenderID As String, bucketID As String) As com.google.firebase.FirebaseOptions
- Initialize (apiKey As String, appID As String, dbUrl As String, GcmsenderID As String, bucketID As String) As com.google.firebase.FirebaseOptions
- Functions:
- FirebaseUI
- Events:
- AccessTokenAvailable (success As Boolean, token As String)
- IdTokenChanged (user As FirebaseUser)
- ResetPassword (success As Boolean)
- SignedIn (user As FirebaseUser)
- SignedOut()
- TokenAvailable (user As FirebaseUser, success As Boolean, token As String)
- UpdateEmail (success As Boolean, email As String)
- UpdatePassword (success As Boolean, password As String)
- UpdateProfile (success As Boolean, request As Object)
- Functions:
- AddIdTokenListener (listener As com.google.firebase.auth.FirebaseAuth.IdTokenListener)
- AddListener (listener As com.google.firebase.auth.FirebaseAuth.AuthStateListener)
- CreateIdTokenListener As com.google.firebase.auth.FirebaseAuth.IdTokenListener
- CreateListener As com.google.firebase.auth.FirebaseAuth.AuthStateListener
- createSignInIntentBuilder As com.firebase.ui.auth.AuthUI.SignInIntentBuilder
- Initialize (EventName As String, defaultClient As String)
- RemoveIdTokenListener (listener As com.google.firebase.auth.FirebaseAuth.IdTokenListener)
- RemoveListener (listener As com.google.firebase.auth.FirebaseAuth.AuthStateListener)
- sendPasswordResetEmail (email As String)
- SendSigninIntent (signin As Intent)
- signInWithCustomToken (token As String)
- signOut
- updateEmail (newEmail As String)
- updatePassword (newPassword As String)
- AddIdTokenListener (listener As com.google.firebase.auth.FirebaseAuth.IdTokenListener)
- Properties:
- CurrentUser As FirebaseUser [read only]
- LanguageCode As String [write only]
- CurrentUser As FirebaseUser [read only]
- Events:
- FirebaseUser
- Functions:
- isAnonymous As Boolean
- IsInitialized As Boolean
- sendEmailVerification
- isAnonymous As Boolean
- Properties:
- DisplayName As String [read only]
- Email As String [read only]
- PhoneNumber As String [read only]
- PhotoUrl As String [read only]
- Uid As String [read only]
- DisplayName As String [read only]
- Functions:
- GoogleBuilder
- Functions:
- BARef As GoogleBuilder
- build As com.firebase.ui.auth.AuthUI.IdpConfig
- DefaultWebClient (client As String) As GoogleBuilder
- setScopes (scopes As java.util.List) As GoogleBuilder
Set the scopes that your app will request when using Google sign-in. See all <a
href="https://developers.google.com/identity/protocols/googlescopes">available
scopes</a>.
scopes: additional scopes to be requested - setSignInOptions (options As com.google.android.gms.auth.api.signin.GoogleSignInOptions) As GoogleBuilder
Set the {@link GoogleSignInOptions} to be used for Google sign-in. Standard
options like requesting the user's email will automatically be added.
options: sign-in options
- BARef As GoogleBuilder
- Properties:
- Params As android.os.Bundle [read only]
- ProviderId As String [write only]
- Params As android.os.Bundle [read only]
- Functions:
- SignInIntentBuilder
- Functions:
- addProvider (provider As com.firebase.ui.auth.AuthUI.IdpConfig)
- build As Intent
- clearProvider
- getIdpGOOGLE_PROVIDER (client As String) As com.firebase.ui.auth.AuthUI.IdpConfig
client:
Return type: @return:config - GetIdpGOOGLE_PROVIDER2 (client As String, scopes As String()) As com.firebase.ui.auth.AuthUI.IdpConfig
- GetIdpGOOGLE_PROVIDER3 (client As String) As com.firebase.ui.auth.AuthUI.IdpConfig
- getResourceId (type As String, resourceName As String) As Int
- Initialize (EventName As String, builder As com.firebase.ui.auth.AuthUI.SignInIntentBuilder)
- IsInitialized As Boolean
- setAlwaysShowSignInMethodScreen (alwaysShow As Boolean) As SignInIntentBuilder
- setAvailableProviders (providers As java.util.List) As SignInIntentBuilder
- setEmailLink (emailLink As String) As SignInIntentBuilder
- setIsSmartLockEnabled (enabled As Boolean) As SignInIntentBuilder
- setIsSmartLockEnabled2 (enableCredentials As Boolean, enableHints As Boolean) As SignInIntentBuilder
- setLogo (logo As Int) As SignInIntentBuilder
- setPrivacyPolicyUrl (privacyPolicyUrl As String) As SignInIntentBuilder
- setTheme (theme As Int) As SignInIntentBuilder
- setTosUrl (tosUrl As String) As SignInIntentBuilder
- addProvider (provider As com.firebase.ui.auth.AuthUI.IdpConfig)
- Properties:
- IdpANONYMOUS_PROVIDER As com.firebase.ui.auth.AuthUI.IdpConfig [read only]
- IdpEMAIL_PROVIDER As com.firebase.ui.auth.AuthUI.IdpConfig [read only]
- IdpFACEBOOK_PROVIDER As com.firebase.ui.auth.AuthUI.IdpConfig [read only]
- IdpGITHUB_PROVIDER As com.firebase.ui.auth.AuthUI.IdpConfig [read only]
- IdpPHONE_VERIFICATION_PROVIDER As com.firebase.ui.auth.AuthUI.IdpConfig [read only]
- IdpTWITTER_PROVIDER As com.firebase.ui.auth.AuthUI.IdpConfig [read only]
- Providerlist As java.util.List [read only]
- IdpANONYMOUS_PROVIDER As com.firebase.ui.auth.AuthUI.IdpConfig [read only]
- Functions:
The Library is free to use. You can however donate for it if you want
Note to download the additional JARs and AARs from here:
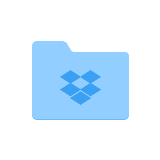
Attachments
Last edited: