The attached program displays the values of the different sensors:
It creates a PhoneSensors object for each type of sensor.
As you can see in the attached code, two Maps are used to hold the phone sensors and the other required data. This way there is no need to write any duplicate code.
A better method for finding the orientation values: https://www.b4x.com/android/forum/threads/orientation-and-accelerometer.6647/page-6#post-476271
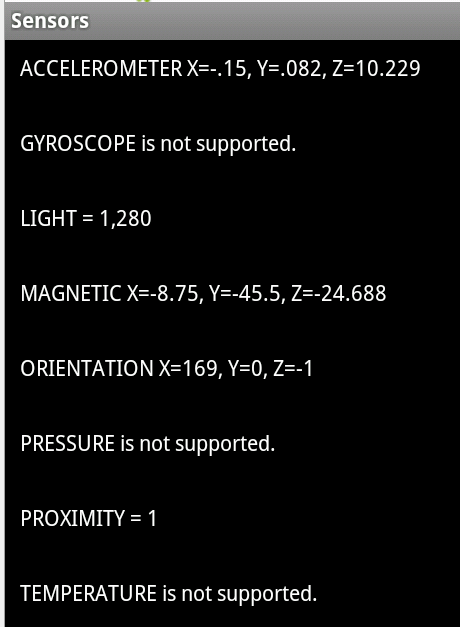
It creates a PhoneSensors object for each type of sensor.
As you can see in the attached code, two Maps are used to hold the phone sensors and the other required data. This way there is no need to write any duplicate code.
A better method for finding the orientation values: https://www.b4x.com/android/forum/threads/orientation-and-accelerometer.6647/page-6#post-476271
Attachments
Last edited: