A simple example with a tank that moves and shoots.
The interesting point in this example is the usage of RevoluteJoint to move and control the cannon.
1. Limits are set to limit the cannon movement.
2. The joint motor is enabled all the time and we just need to change the target speed to move or hold the cannon:
B4X:
If UpDown Then
revjoint.MotorSpeed = 1
Else If DownDown Then
revjoint.MotorEnabled = True
revjoint.MotorSpeed = -1
Else
revjoint.MotorSpeed = 0
Kane.Body.AngularVelocity = 0
End If
By setting the MotorSpeed to 0 the joint motor prevents the cannon from moving when forces act on the tank.
Code to fire a cannon ball:
B4X:
Private Sub Fire
Dim template As X2TileObjectTemplate = TileMap.GetObjectTemplateByName(ObjectLayer, "bullet")
template.BodyDef.Position = Kane.Body.Position
'set the velocity direction based on the cannon direction.
template.BodyDef.LinearVelocity = Kane.Body.Transform.MultiplyRot(X2.CreateVec2(30, 0))
'better simulation of fast moving objects
template.BodyDef.Bullet = True
TileMap.CreateObject(template)
End Sub
To prevent the bullet from hitting the "kane" object the bullet category bits is set to 2 and the kane mask bits are set to 65533 (65535 - 2). Category bits must be a power of 2.
As usual all the game objects are defined with a tiled map:
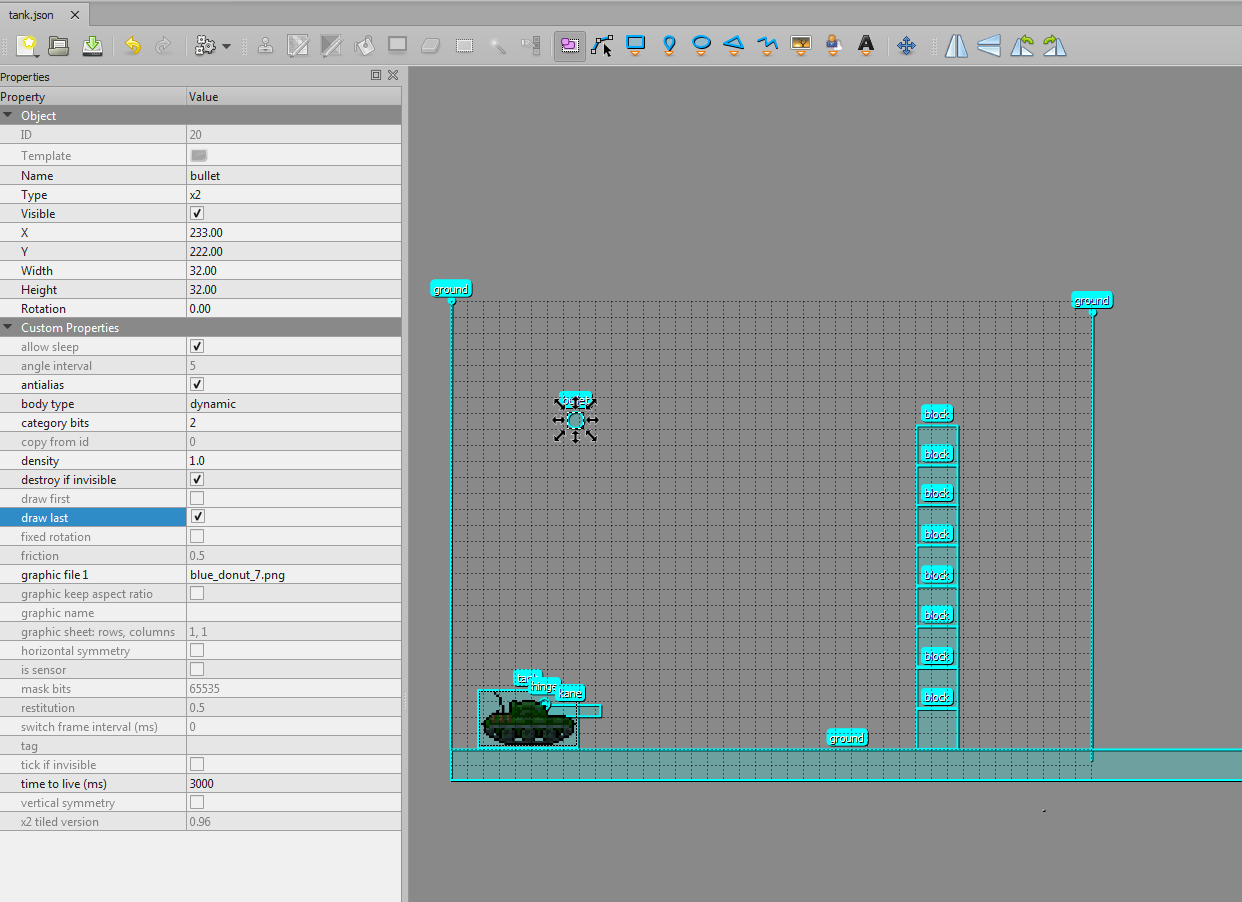
If you don't see the custom properties in the Tiled editor then you need to import the custom properties.
The example is included in the example pack.
https://www.b4x.com/android/forum/threads/xui2d-example-pack.96454/