This is a very early demonstration of a new feature that is coming to B4X:
Code (no timers!):
The purpose of this feature is to make it simpler to work with asynchronous methods.
Instead of making a call and then waiting for the result in a different sub you will be able to implement all the logic as if it was non-asynchronous.
This feature is similar to stackless coroutines or async / await in other programming languages.
It is not similar to a loop with DoEvents as the main thread is not caught in the sub. The sub context is stored and is later resumed.
Code (no timers!):
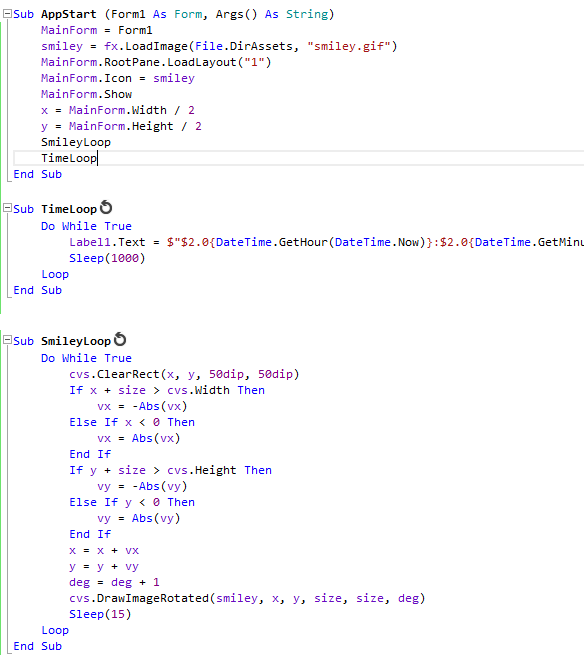
The purpose of this feature is to make it simpler to work with asynchronous methods.
Instead of making a call and then waiting for the result in a different sub you will be able to implement all the logic as if it was non-asynchronous.
B4X:
'pseudo code
Sub Download
Job.Download(...)
Wait For JobDone
If Job.Success Then Log(Job.GetString)
End Sub
This feature is similar to stackless coroutines or async / await in other programming languages.
It is not similar to a loop with DoEvents as the main thread is not caught in the sub. The sub context is stored and is later resumed.
Last edited: