The ScrollView is a very useful container which allows you to show many other views on a small screen.
The ScrollView holds an inner panel view which actually contains the other views.
The user vertically scrolls the inner panel as needed.
In this example we will load images from the sdcard and add those to a ScrollView.
Adding views to a ScrollView is done by calling:
In order to avoid "out of memory" errors we use LoadBitmapSample instead of LoadBitmap. LoadBitmapSample accepts two additional parameters: MaxWidth and MaxHeight. When it loads a bitmap it will first get the bitmap original dimensions and then if the bitmap width or height are larger than MaxWidth or MaxHeight the bitmap will be subsampled accordingly. This means that the loaded bitmaps will have lower resolution than the original bitmap. The width / height ratio is preserved.
The following code sets the inner panel height and adds an ImageView for each loaded bitmap:
The code that is loading the bitmaps looks for jpg files under /sdcard/Images
If you want to run this program on the emulator you will first need to create this folder and copy some images to it.
This is done with the "adb" command, that comes with Android SDK.
Open a shell console (Windows Start - Run - Cmd).
Go to the sdk tools folder and issue:
The -e parameter tells adb to send the command to the only connected emulator.
The command is mkdir with the name of the folder.
Note that Android file system is case sensitive.
Now we need to copy some images to this folder.
This is done with the push command:
This will copy all files under c:\temp to the Images folder.
The emulator is very slow compared to a real device. While on a real device 50 large images load in 2-3 seconds. It can take a long time for the emulator to load a few small images. I recommend you to copy 2 - 3 small images at most.
Also the experience of the ScrollView on the emulator cannot be compared to a real device (with capacitive screen).
The program is available here: http://www.b4x.com/android/files/tutorials/ScrollView.zip
The ScrollView holds an inner panel view which actually contains the other views.
The user vertically scrolls the inner panel as needed.
In this example we will load images from the sdcard and add those to a ScrollView.
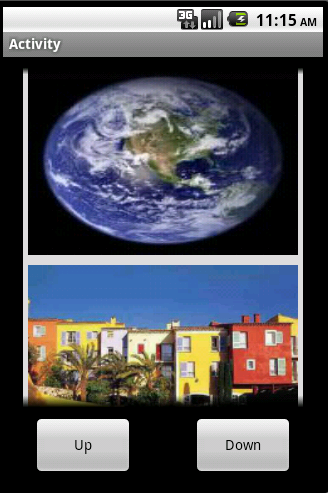
Adding views to a ScrollView is done by calling:
B4X:
ScrollView.Panel.AddView(...)
The following code sets the inner panel height and adds an ImageView for each loaded bitmap:
B4X:
ScrollView1.Panel.Height = 200dip * Bitmaps.Size 'Set the inner panel height according to the number of images.
For i = 0 To Bitmaps.Size - 1
Dim iv As ImageView 'create an ImageView for each bitmap
iv.Initialize("") 'not interested in any events so we pass empty string.
Dim bd As BitmapDrawable
bd.Initialize(Bitmaps.Get(i))
iv.Background = bd 'set the background of the image view.
'add the image view to the scroll bar internal panel.
ScrollView1.Panel.AddView(iv, 5dip, 5dip + i * 200dip, ScrollView1.Width - 10dip, 190dip)
Next
If you want to run this program on the emulator you will first need to create this folder and copy some images to it.
This is done with the "adb" command, that comes with Android SDK.
Open a shell console (Windows Start - Run - Cmd).
Go to the sdk tools folder and issue:
B4X:
c:\android-sdk-windows\tools> adb -e shell mkdir /sdcard/Images
The command is mkdir with the name of the folder.
Note that Android file system is case sensitive.
Now we need to copy some images to this folder.
This is done with the push command:
B4X:
adb -e push "C:\temp" /sdcard/Images
The emulator is very slow compared to a real device. While on a real device 50 large images load in 2-3 seconds. It can take a long time for the emulator to load a few small images. I recommend you to copy 2 - 3 small images at most.
Also the experience of the ScrollView on the emulator cannot be compared to a real device (with capacitive screen).
The program is available here: http://www.b4x.com/android/files/tutorials/ScrollView.zip